PanOS REST API is an incredibly powerful tool to manage both Palo Alto Firewalls and Panorama through various API platforms such as Postman, Python or even CLI. Using the API for a while, I find it extremely useful especially working with larger configuration files. You can easily scrape through the entire configuration tree and find only the elements that you need.
You might think that the CLI and Web-GUI already provide robust management to the firewall so, why do I need another option? In this blog post, we will go through the PanOS REST API fundamentals and some of the use cases.
What is a REST API?
An API, or Application Programming Interface, is a set of rules and protocols for building and interacting with software applications. It defines the methods and data structures that developers can use to interact with the software programmatically, rather than through a user interface.
A REST API, or Representational State Transfer API, is a type of API that adheres to the principles of REST architectural style. It uses standard HTTP methods like GET, POST, PUT, and DELETE for operations. REST APIs are designed to be stateless, meaning that each request from a client to the server must contain all the information needed to understand and complete the request.
In the context of a REST API, a URI, or Uniform Resource Identifier, is the address used to access a resource on a server. It allows clients to identify and interact with resources using specific URLs, where each URL returns data related to a particular aspect of the application.
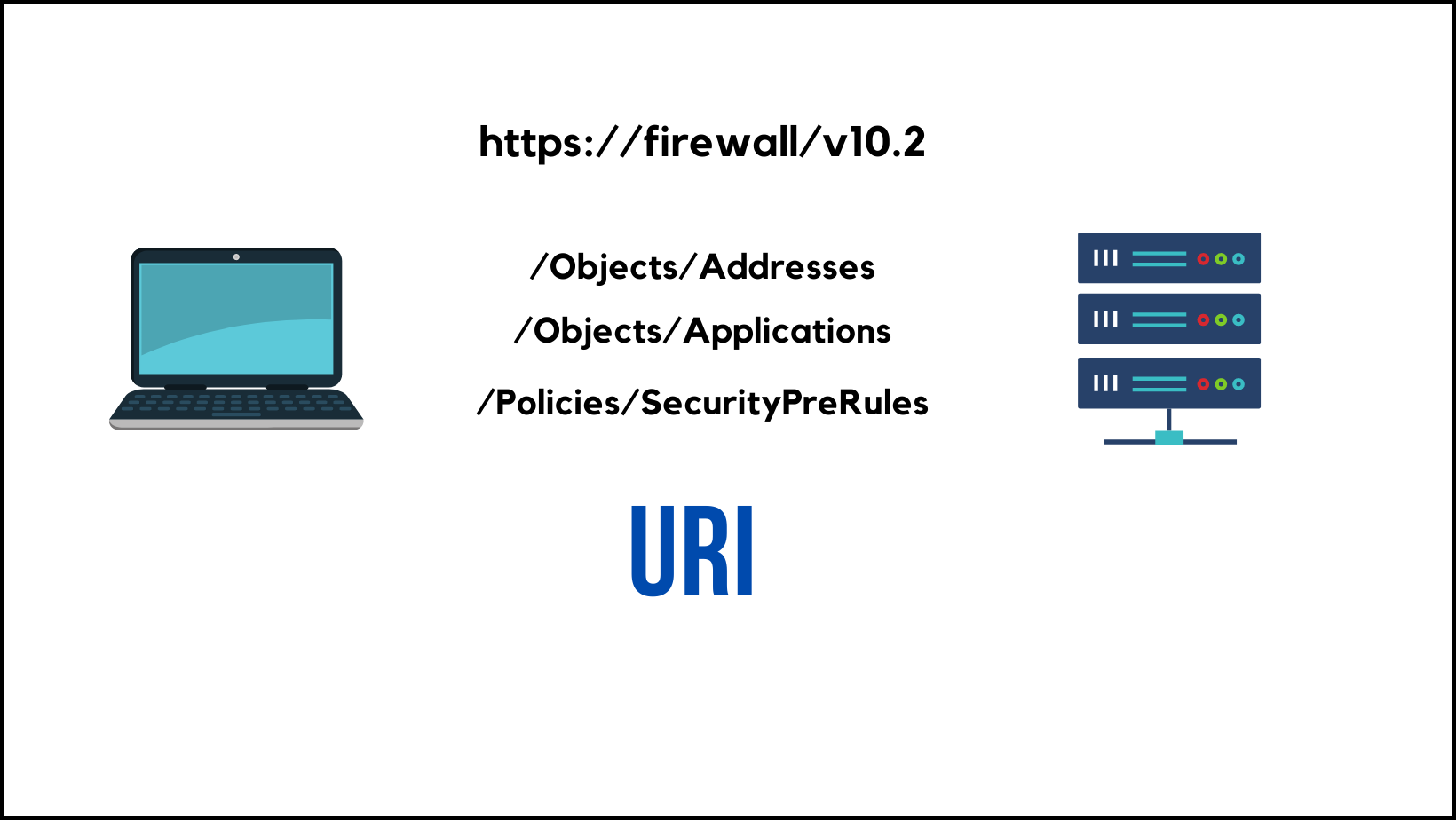
The XML API uses a tree of XML nodes to map firewall or Panorama functionality. To make an API request, you can specify the XPath to the XML node that corresponds to a specific setting. XPath allows you to navigate through the hierarchical XML tree structure of the firewall.
REST API on the other hand uses CRUD (Create, Read, Update and Delete) to view, create, delete or make changes to the firewall/panorama configurations.
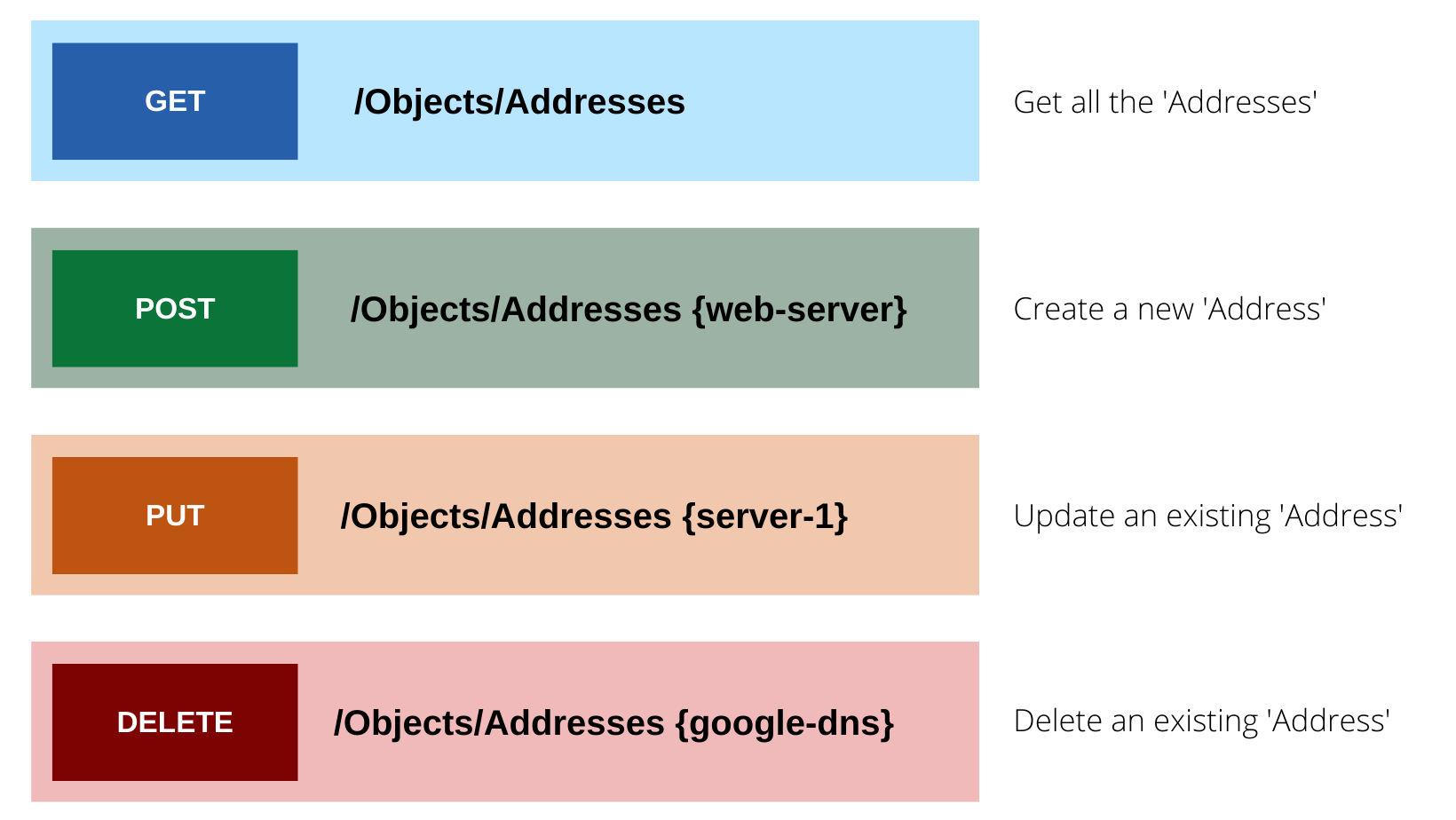
Getting started with PanOS REST API
API Key
To access the API (XML or REST), we need to enable API access for the firewall administrators and get the API key. If the Admins are part of the built-in roles such as 'superuser', they should already have access to the REST API and we just need to generate the API Key. It is recommended to use a dedicated user account for API access and provide the least amount of access.
For this example, I'm going to create an 'admin-role' with only access to the REST API and associate an 'Admin' account with it.
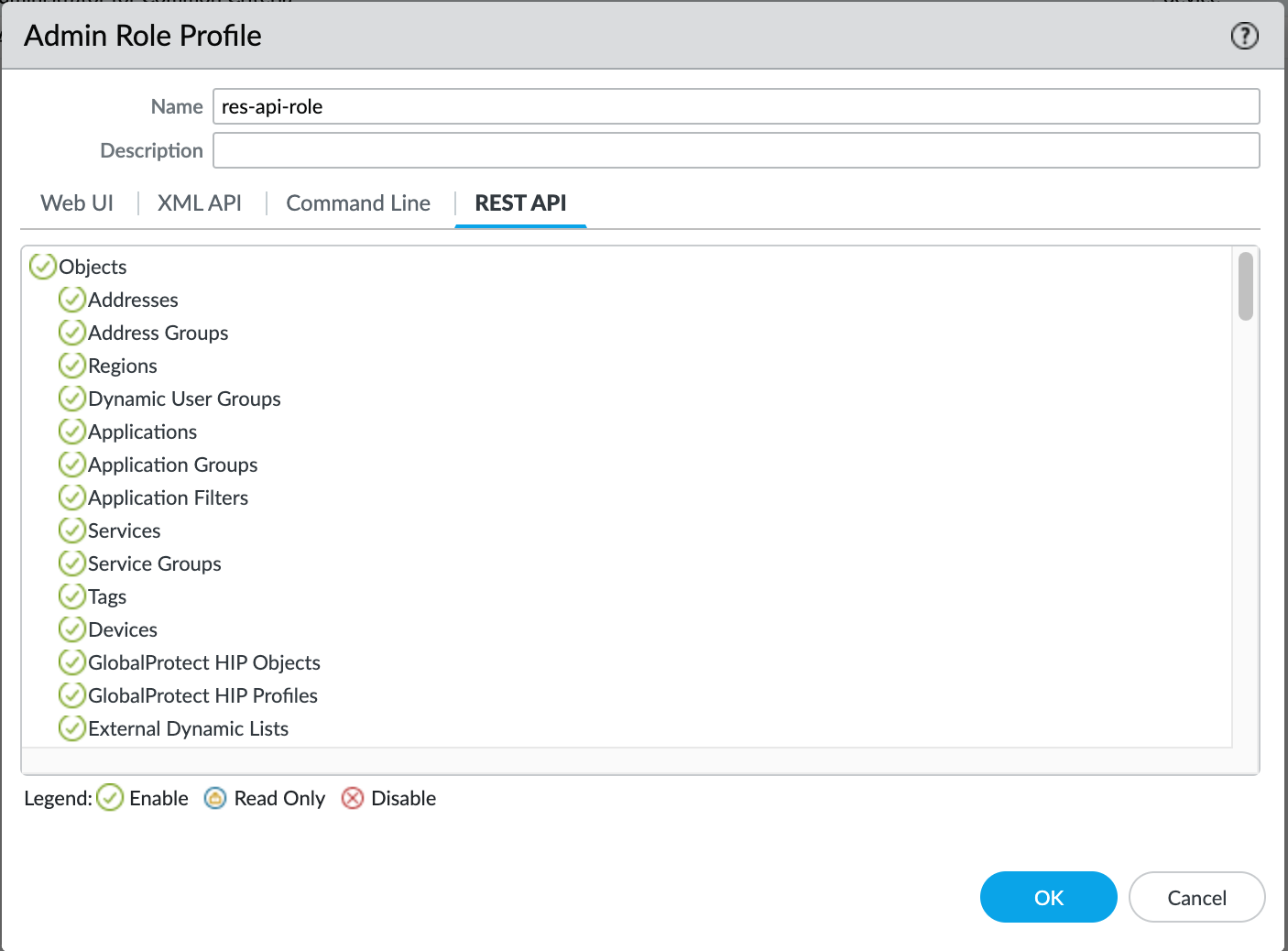
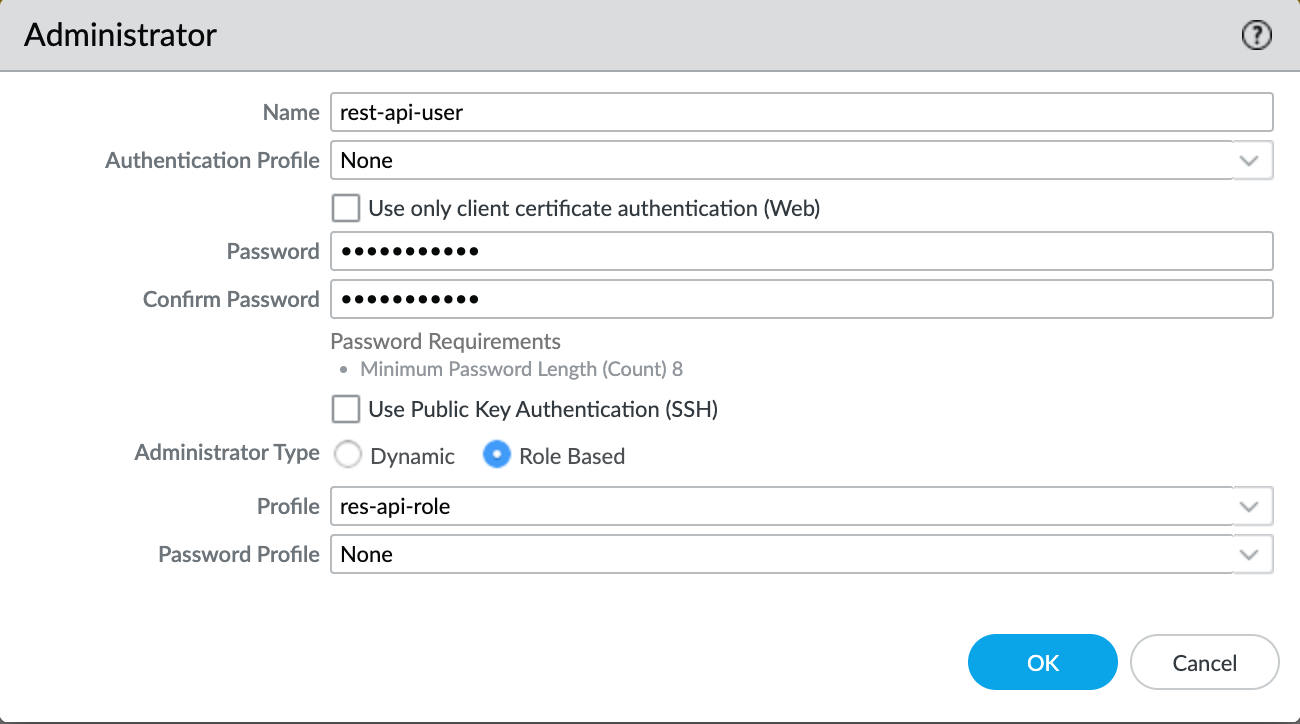
You can generate the API Key by making a GET or POST request to the firewall using the following syntax, curl -k -X GET 'https://<firewall>/api/?type=keygen&user=<username>&password=<password>'
suresh@mac:~|⇒ curl -k -X GET 'https://palo-lab.local/api/?type=keygen&user=rest-api-user&password=Password123'
<response status = 'success'><result><key>LUFRPT1ueFpaNXFCUGM2ckxXNGFIeVRscVkrL21tZHM9aldDN1pQMUxMN0FDRCtIa0tUczBSbHhlTDZtZWFUb1F4d3FhSnpRTVVwS3VsYXlxblODJFS2xWczUwL3FlRQ==</key></result></response>%
Please copy the API key and store it in a secure place such as a Password Manager. We will use this Key throughout the examples.
If you want to retrieve the key using Python, here is how to do it
import requests
import xml.etree.ElementTree as ET
query = {'type':'keygen', 'user':'rest-api-user', 'password':'Password123'}
response = requests.get('https://palo-lab.local/api', params=query, verify=False)
# Parse the XML string
root = ET.fromstring(response.text)
# Find the key element and get its text
api_key = root.find(".//key").text
print(api_key)
REST API Document
You can access the REST API documentation by navigating to the following URL, https://FIREWALL_IP/restapi-doc
as shown below. You can explore the different URIs, query parameters and body requirements available to make a successful API call.
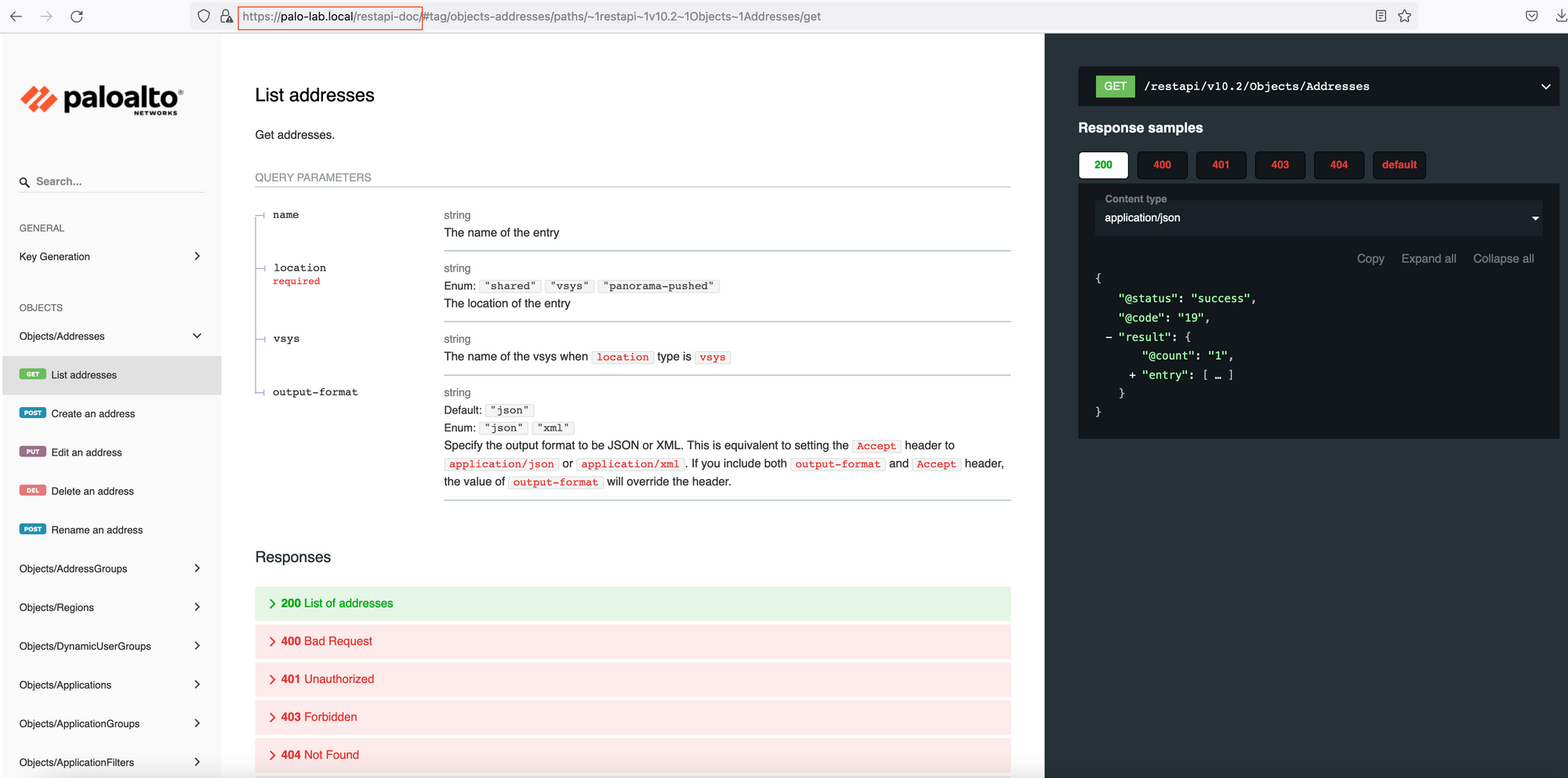
First API Call
By default, the firewall and Panorama support API requests over HTTPS. To authenticate the API request to the firewall or Panorama, provide the API key in any of the following ways:
- Use the custom HTTP header, X-PAN-KEY: <key> to include the API key in the HTTP header.
- For the XML API, include the API key as a query parameter in the HTTP request URL. (not applied to REST API)
GET - Address / Objects
Let's make a GET request to retrieve all the Address Objects configured on the firewall. I'm using Postman to send the API calls however, you can choose whichever platform is available to you.
The API Doc below shows all the information we need to make the API call which are the URL, URI and query parameters.
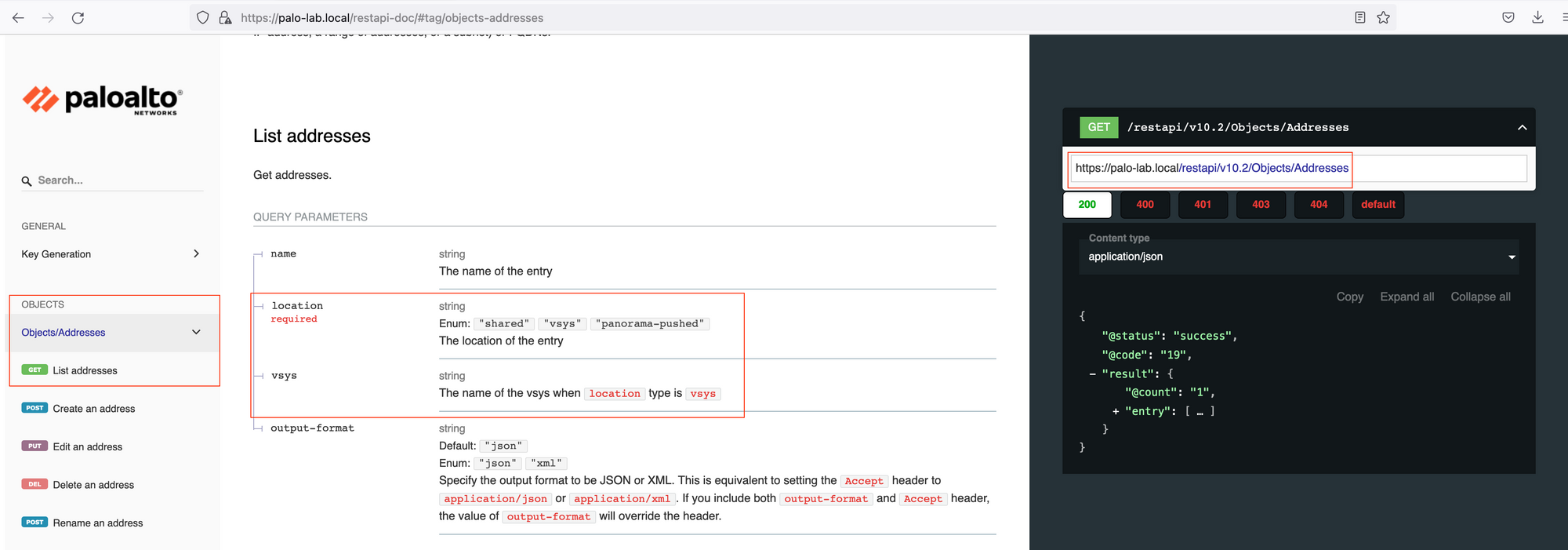
Location
is the mandatory query parameter, since we are connecting to the Firewall, the location will be the vsys
. If you are connecting to the Panorama, the location would become device-group
.
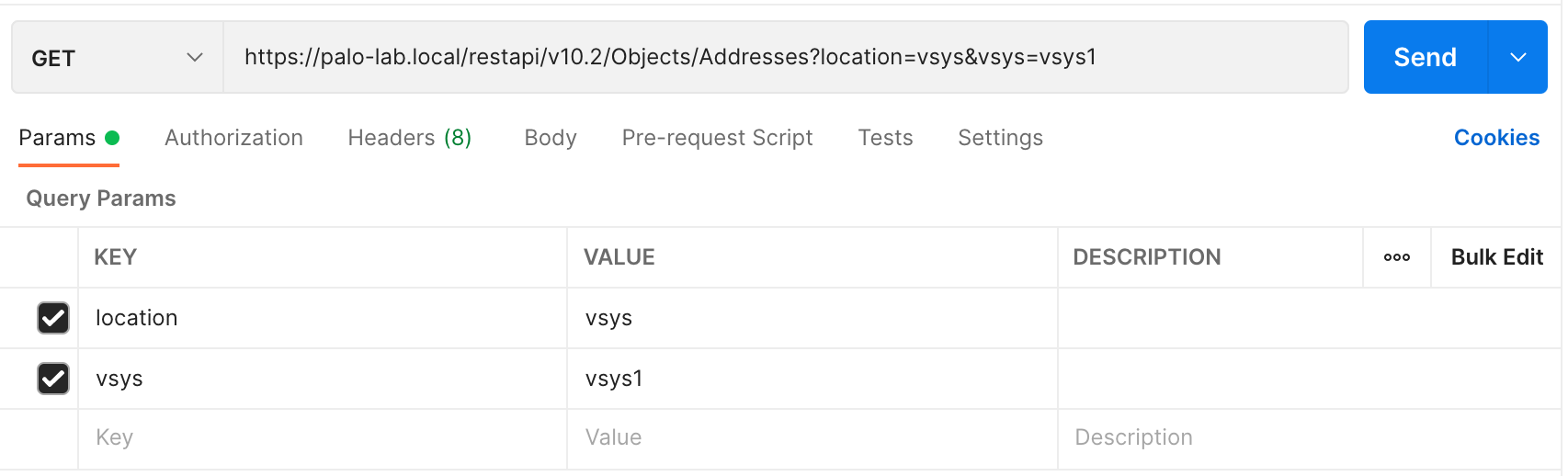
I'm also specifying the API key within the Headers as shown below.
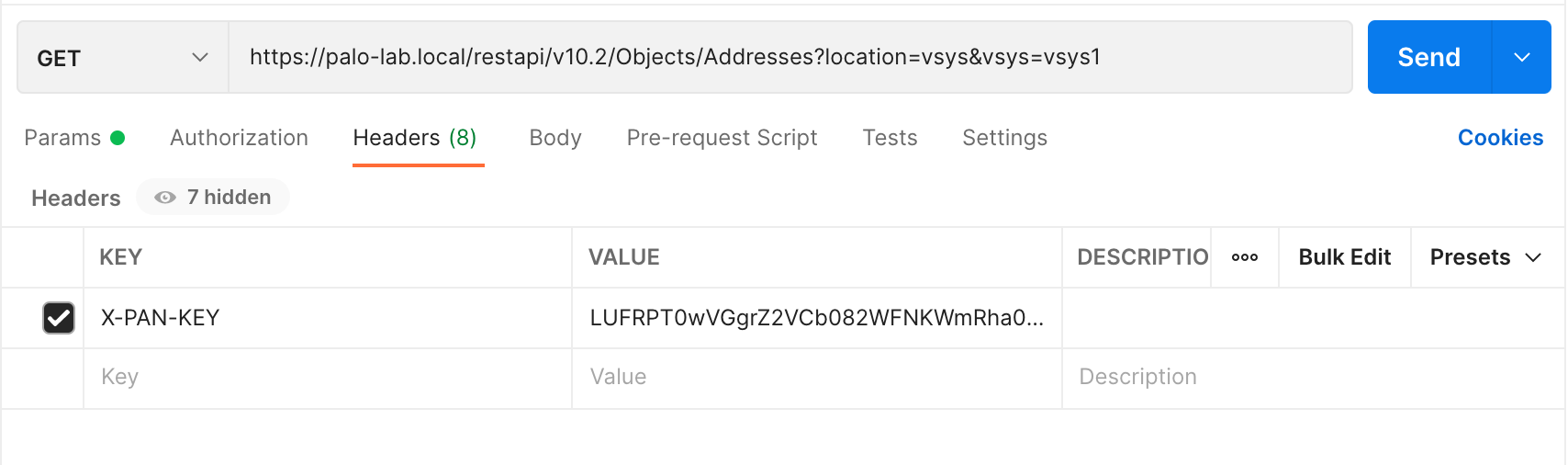
Once you hit 'send', you will get a JSON output which contains all the objects currently configured on the firewall.
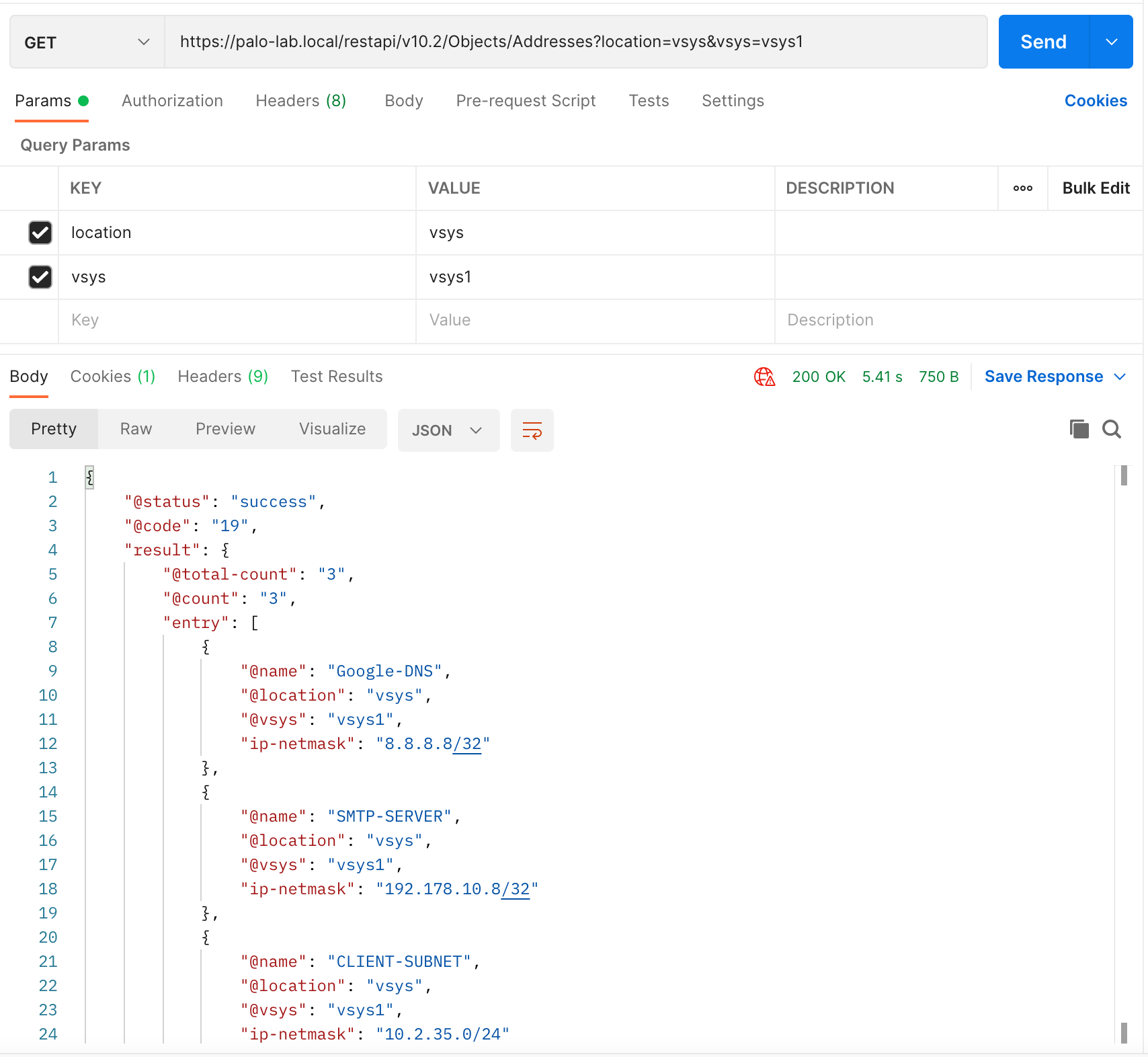
CURL
Alternatively, you can use the CURL command to make the API call as shown below. My personal preference is to use Postman whenever possible.
curl -X GET 'https://palo-lab.local/restapi/v10.2/Objects/Addresses?location=vsys&vsys=vsys1' -H 'X-PAN-KEY: LUFRPT0wVGgrZ2VCb082WFNKWmRha0puUnZodE9sWXc9aldDN1pQMUxMN0FDRCtIa0tUczBSbHhlTDZtZWFUb1F4d3FhSnpRTVVwS2ZaK0I0N2pTVE5TaXhQQndPbysxeQ==' -k | jq
{
"@status": "success",
"@code": "19",
"result": {
"@total-count": "3",
"@count": "3",
"entry": [
{
"@name": "Google-DNS",
"@location": "vsys",
"@vsys": "vsys1",
"ip-netmask": "8.8.8.8/32"
},
{
"@name": "SMTP-SERVER",
"@location": "vsys",
"@vsys": "vsys1",
"ip-netmask": "192.178.10.8/32"
},
{
"@name": "CLIENT-SUBNET",
"@location": "vsys",
"@vsys": "vsys1",
"ip-netmask": "10.2.35.0/24"
}
]
}
}
POST - Address / Objects
We can also use the API to create (POST) various resources on the firewall. The following example shows how to create an Address Object via the API.
One of the additional parameters required for POST is the Name of the Object. The body of the request should be JSON as shown below. You can find all of the required parameters from the restapi-doc URL I mentioned above.
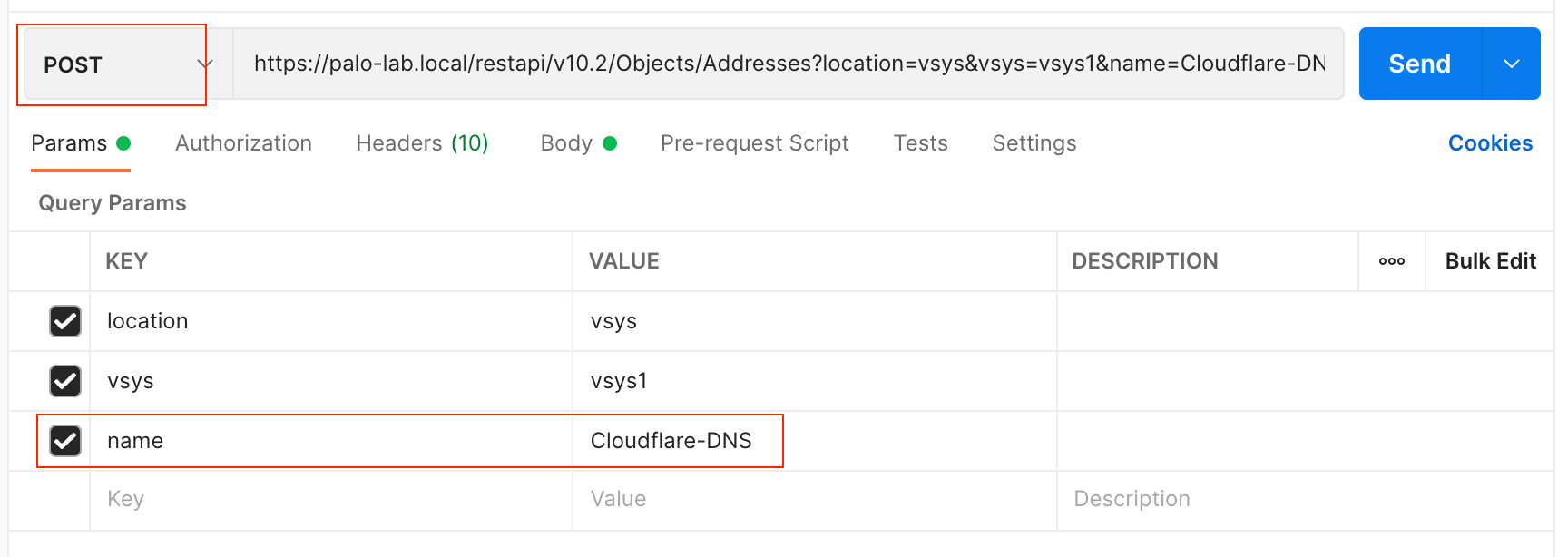
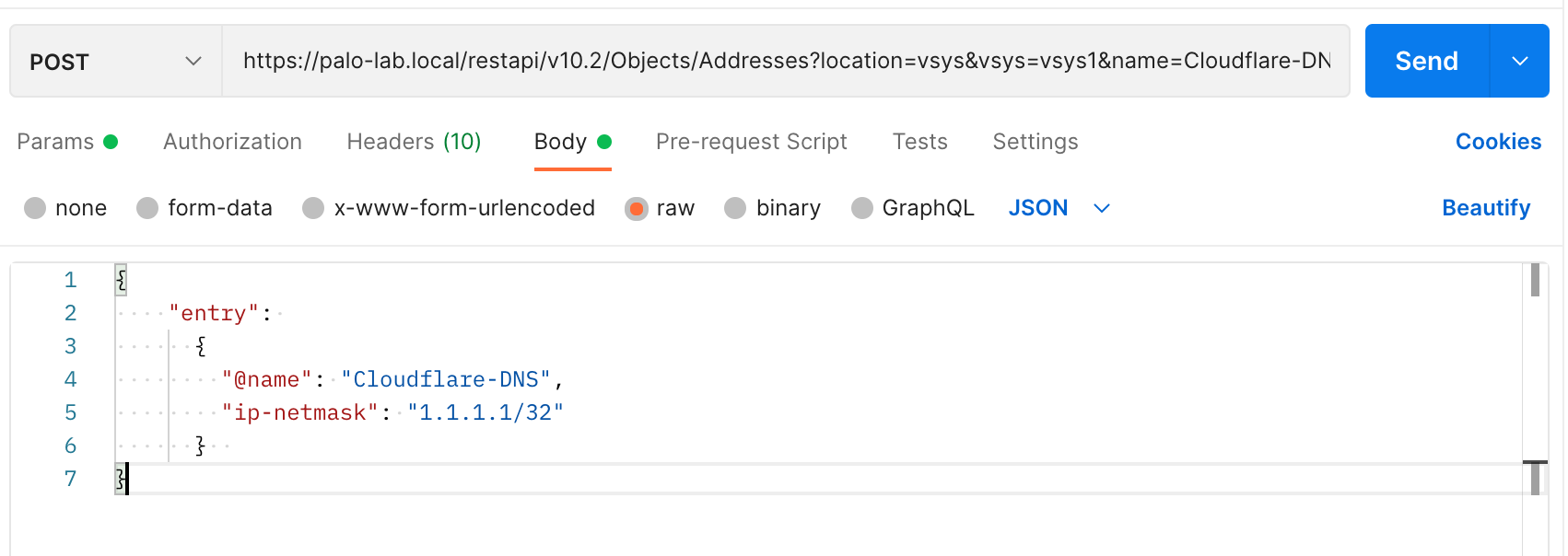
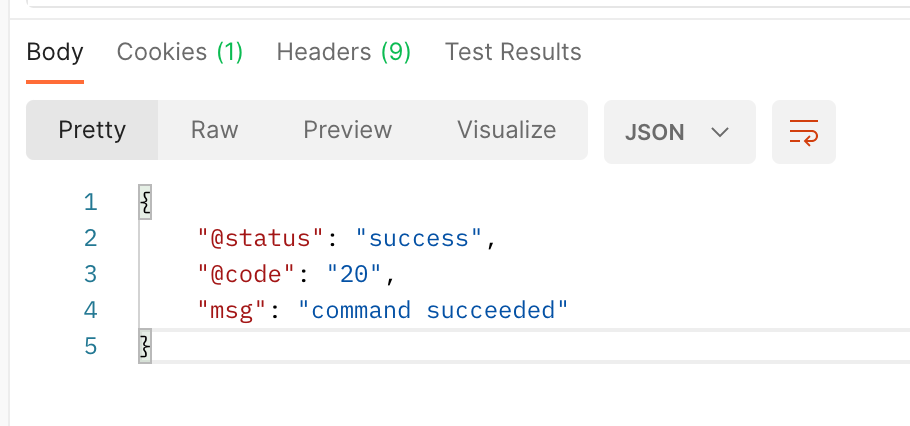
As you can see below, the Object has been created.
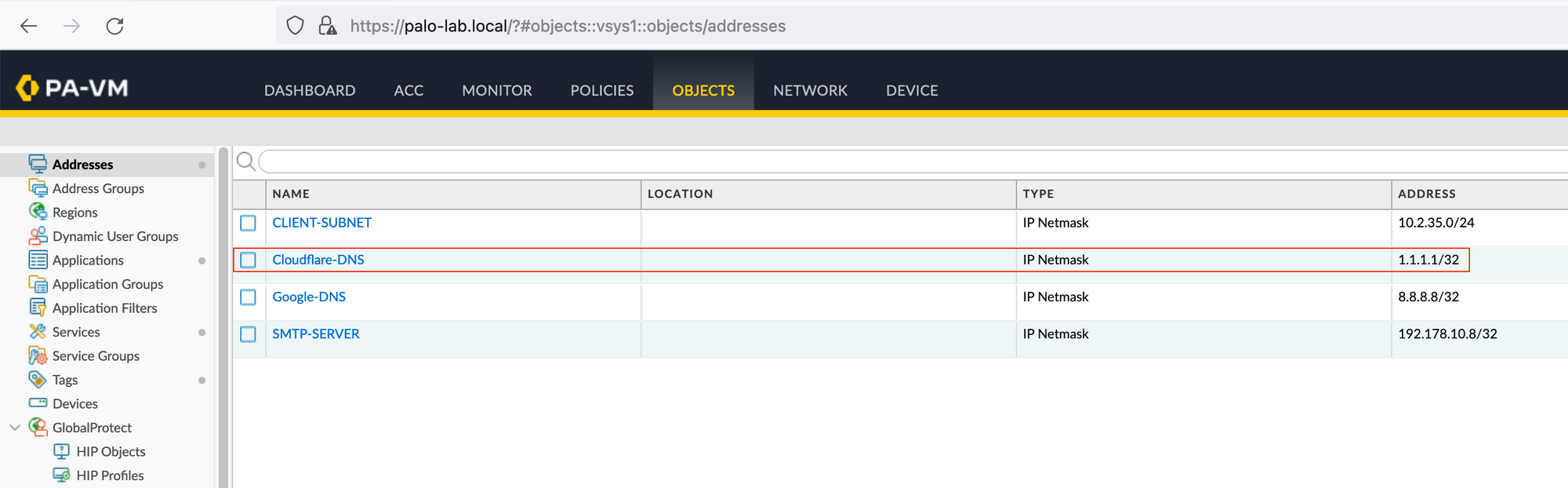
If you try and re-send the POST request, you will get a message indicating that the exact same object already exists.
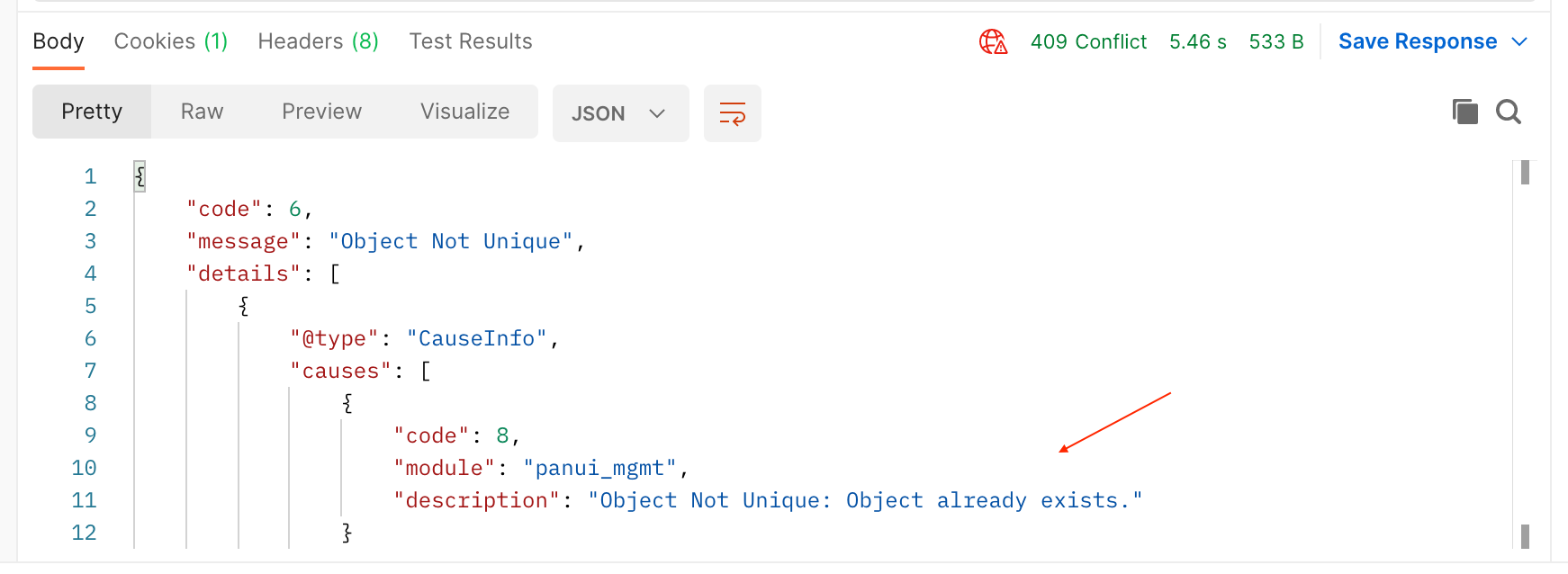
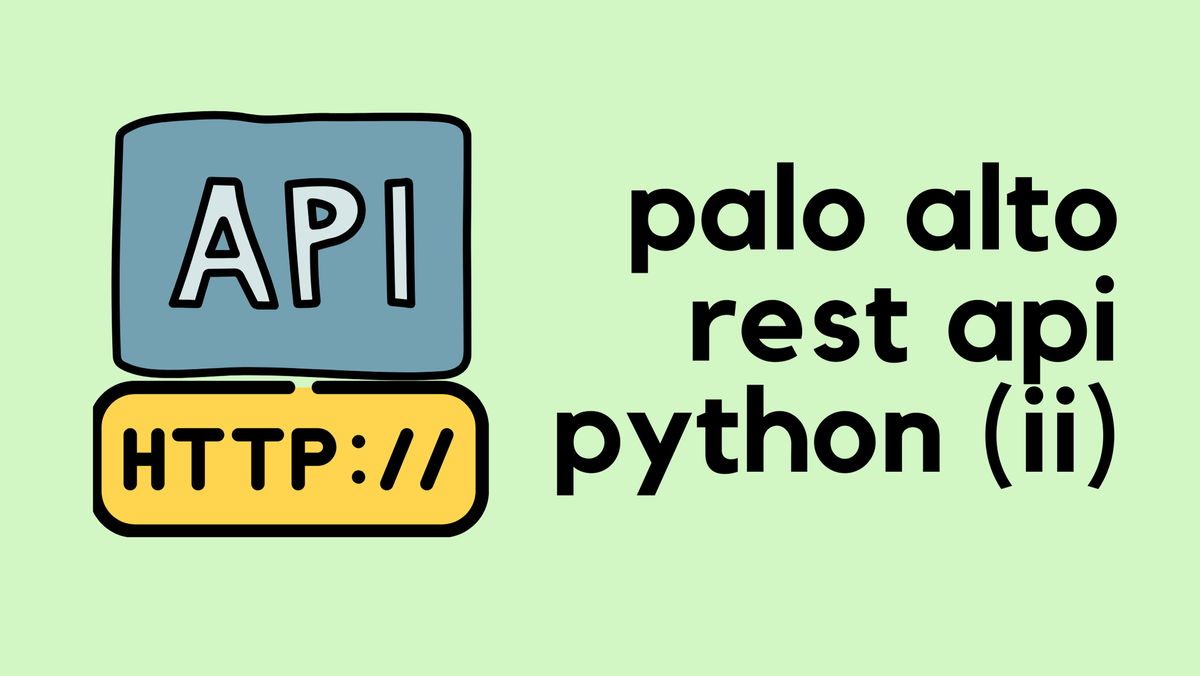