If you are working with firewalls on a daily basis, at some point you are going to come across having to create multiple address objects at once. Of course, it wouldn't make sense to create them one by one so, you need to find a way to automate the creation of such objects. In this blog post, we will look into how to configure bulk address objects on the Palo Alto firewalls using the PanOS REST API and Python.
If you are not familiar with the PanOS REST API, I highly recommend checking out my previous posts here.
Create a Single Address Object
Let's start from the basics and configure an Address Object using the API. I'm going to use the Python Requests module to make the API call. When you create an address object, you need to provide two mandatory pieces of information that are object name and ip-netmask. I've configured them as key-value pairs inside the JSON body object.
import requests
import json
# Disable self-signed warning
requests.packages.urllib3.disable_warnings()
location = {'location': 'device-group', 'device-group': 'lab', 'name': 'google_dns'}
headers = {'X-PAN-KEY': 'YOUR_API_KEY'}
api_url = "https://Firewall_IP/restapi/v10.2/Objects/Addresses"
body = json.dumps(
{
"entry":
{
"@name": "google_dns",
"ip-netmask": "8.8.8.8",
}
}
)
r = requests.post(api_url, params=location, verify=False, headers=headers, data=body)
print(r.text)
When you run the script, you will see the below message as an output which indicates that the object has been created successfully. You can also log in to the firewall to verify that the new object indeed exists.
{"@status":"success","@code":"20","msg":"command succeeded"}
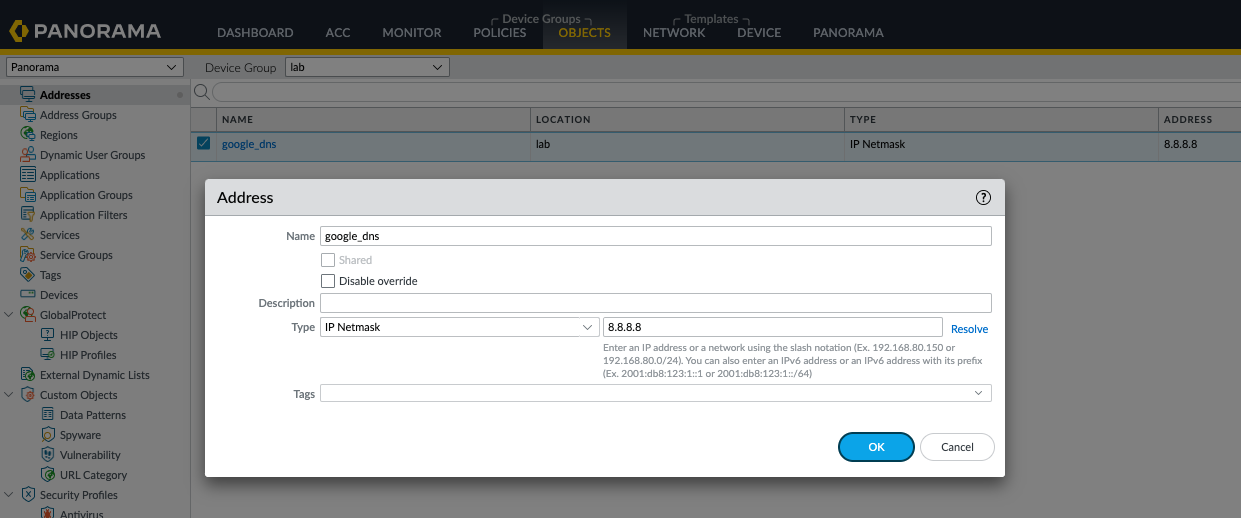
Create Multiple Address Objects using a Python Dictionary
Now that we are comfortable creating a single object, let's expand on it and create multiple objects using a Python dictionary. First, I've created a list called objects
and then created two items inside the list.
Each item in the list is a Python dictionary that holds information about a specific address object. Finally, we can use a for loop
to iterate over the list and create all the objects inside the list.
import requests
import json
# Disable self-signed warning
requests.packages.urllib3.disable_warnings()
objects = [
{
"name": "server_1",
"ip": "192.168.10.10"
},
{
"name": "server_2",
"ip": "192.168.10.11"
}
]
headers = {'X-PAN-KEY': 'API_KEY'}
api_url = "https://Firewall_IP/restapi/v10.2/Objects/Addresses"
for object in objects:
location = {'location': 'device-group', 'device-group': 'lab', 'name': object['name']}
body = json.dumps(
{
"entry":
{
"@name": object['name'],
"ip-netmask": object['ip']
}
}
)
r = requests.post(api_url, params=location, verify=False, headers=headers, data=body)
print(r.text)

Create Multiple Address Objects using CSV File
Python dictionary is useful to create just a few addresses but ideally, we want to use something like a CSV file that holds the information about the address objects.
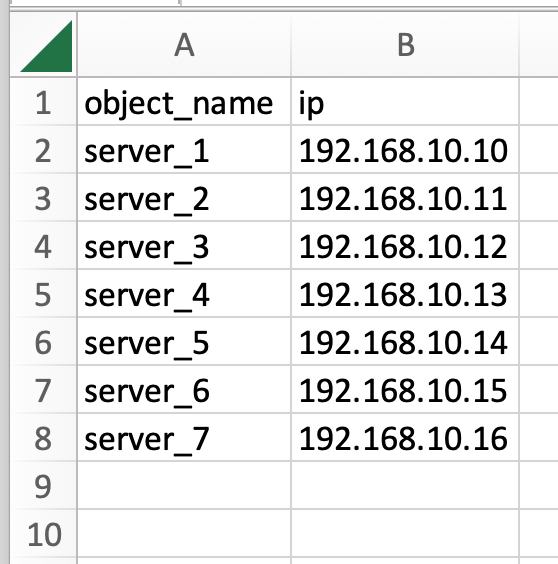
Since we are working with CSV files, we need to import the Python csv
library. We can then use the csv.DictReader()
class to read each row and pass that information to the API call.
Finally, we can use a for loop
to iterate over each row of the CSV file and configure the address objects on the firewall.
import requests
import json
import csv
# Disable self-signed warning
requests.packages.urllib3.disable_warnings()
headers = {'X-PAN-KEY': 'API_KEY'}
api_url = "https://Firewall_IP/restapi/v10.2/Objects/Addresses"
with open ('object_source.csv') as f:
reader = csv.DictReader(f)
for row in reader:
name = row['object_name']
ip = row['ip']
location = {'location': 'device-group', 'device-group': 'lab', 'name': name}
body = json.dumps(
{
"entry":
{
"@name": name,
"ip-netmask": ip
}
}
)
r = requests.post(api_url, params=location, verify=False, headers=headers, data=body)
print(r.text)
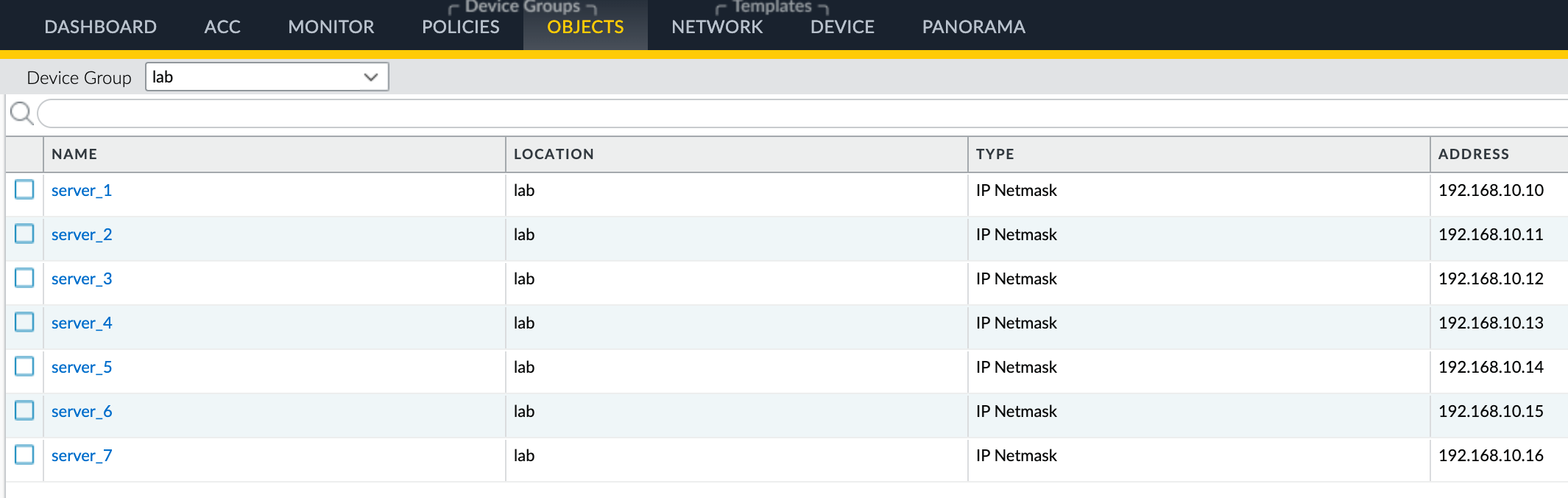
Closing thoughts
There are many ways you can bulk-create address objects but my preference has always been REST API. Please let me know in the comments how you prefer to create multiple objects.
If you want to learn how to use the pan-os-python Library to bulk create/edit objects, please check out my other blog post below.
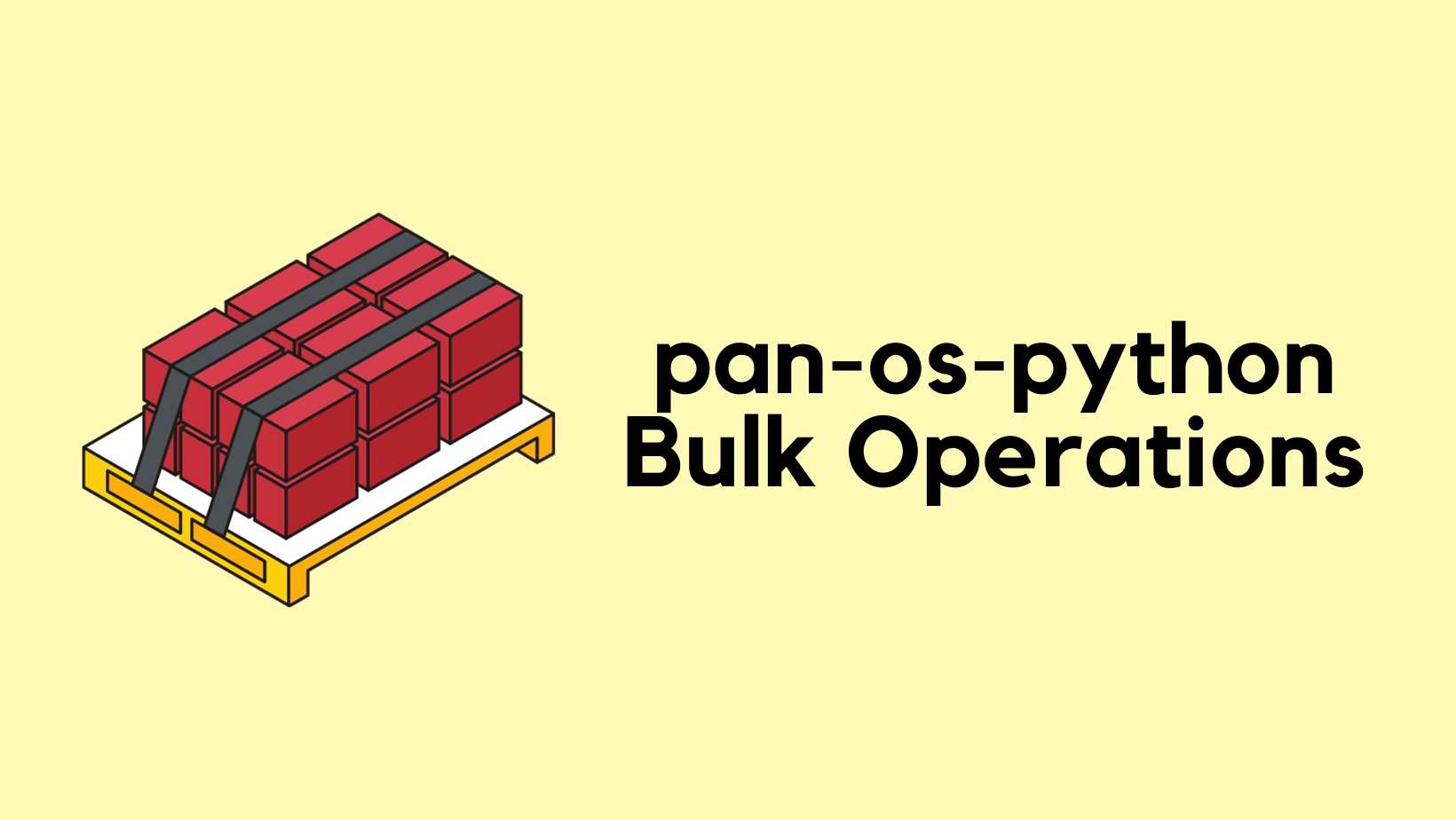