In this blog post, we're diving into a simple Python script. This script will SSH into your network devices and back up their configuration directly to your computer. We're purposely keeping it basic - no added complications of date/time stamps, different vendors or error-handling or running parallel operations.
The goal here isn't to present you with a highly advanced script, but rather to offer a basic, foundational tool. It's a script you can use as is or build upon to make something that fits your specific needs.
Netmiko Overview
We'll be using the Netmiko library in this script to connect to our devices. Netmiko is a Python library designed to simplify the automation of network devices across a wide range of vendors. It provides a unified interface to interact with these devices.
Installing nemiko
is as simple as running the following command.
pip install netmiko
For those of you eager to dive deeper into Netmiko, don't forget to check out my other blog post where I delve into the library's features and uses in greater detail.
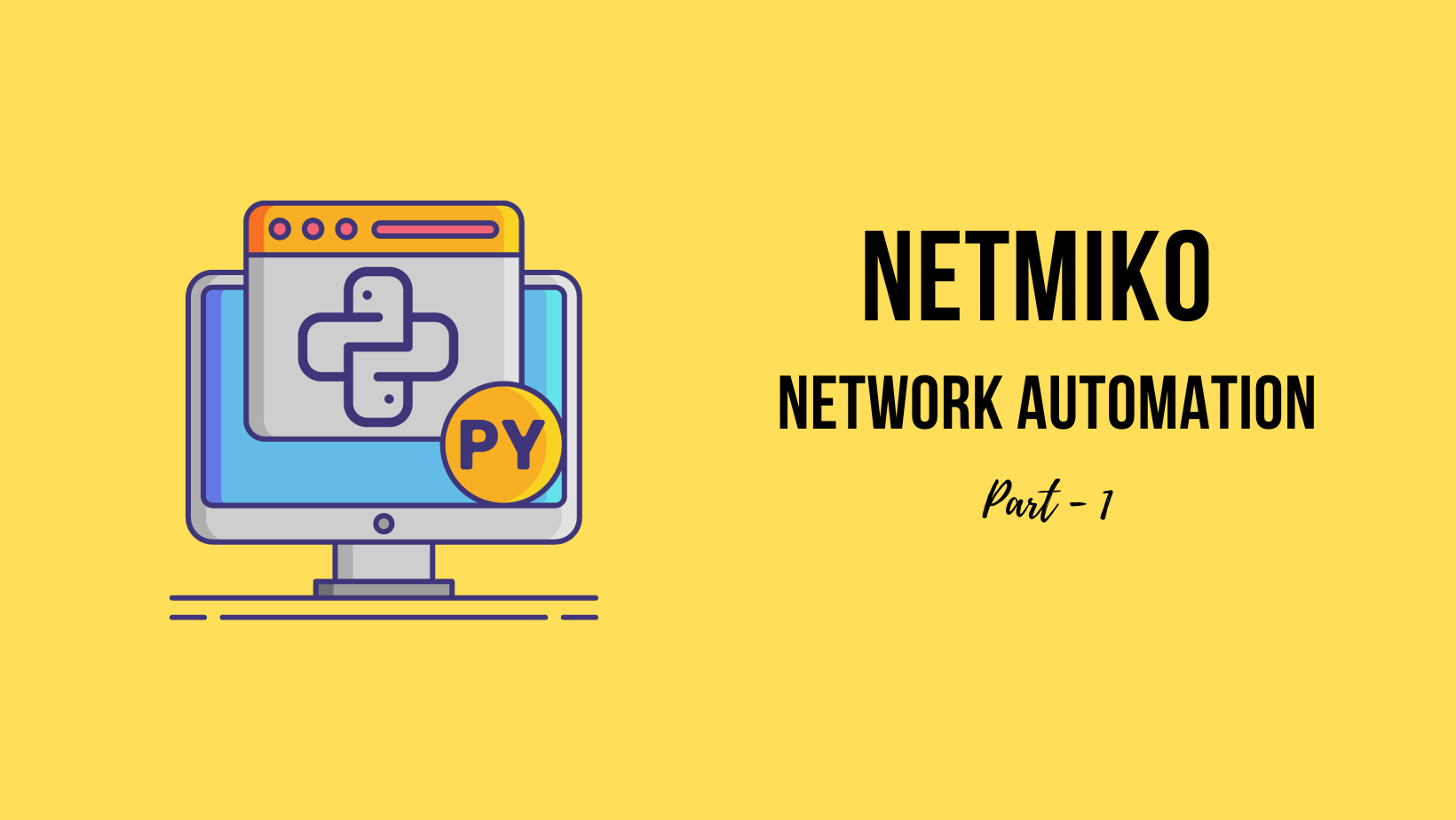
Configuration Backup Script
from netmiko import ConnectHandler
import getpass
passwd = getpass.getpass('Please enter the password: ')
file_dir = '/Users/suresh/Documents/config-backup/backups'
switch_list = ['192.168.10.10', 'core-switch-01', 'test_switch']
device_list = []
for ip in switch_list:
device = {
"device_type": "cisco_ios",
"host": ip,
"username": "suresh",
"password": passwd,
"secret": passwd # Enable password
}
device_list.append(device)
for device in device_list:
host_name = device['host']
connection = ConnectHandler(**device)
show_run = connection.send_command('show run')
with open(f"{file_dir}/show_run_{host_name}.txt", 'w') as f:
f.write(show_run)
connection.disconnect()
- The script starts by importing two modules -
netmiko
, which we use to establish SSH connections to our network devices, andgetpass
, which is a built-in Python module for securely handling password prompts. - It then prompts the user to enter a password using the
getpass.getpass()
function. This function securely captures the user's password input without displaying it on the screen. - Next, it defines the directory where the configuration backups will be saved (
file_dir
) and the list of network devices that the script will connect to (switch_list
). - The script creates an empty list named
device_list
. This list will be populated with dictionaries, each representing a network device. Each dictionary will contain key-value pairs that represent the necessary details for SSH connections (device type, host IP, username, password, and secret/enable password). - It then loops over the IP addresses in
switch_list
, creates a dictionary with the SSH details for each device, and appends it todevice_list
. - With all the SSH details prepared, the script loops over
device_list
, making a SSH connection to each device using theConnectHandler
Class fromnetmiko
. - Once connected, it sends the command
show run
to the device to get the running configuration. The result of this command is stored in the variableshow_run
. - It then creates and opens a new file in the backup directory (
file_dir
) named with the patternshow_run_{host_name}.txt
. The running configuration (show_run
) is then written into this file. - After backing up the configuration, the script disconnects from the device before moving to the next one in the
device_list
.
Enhancements
Our initial script is a solid starting point, but there's plenty of room for enhancements. As you grow more comfortable with Python and network automation, you might consider adding these improvements:
- Concurrency: The script currently connects to each device one by one. To speed up the process, you could introduce concurrency. The
concurrent.futures
module in Python allows us to run the script concurrently. Instead of waiting for each device connection to complete before starting the next, it allows multiple connections to be established and managed simultaneously. - Error handling: At present, if our script fails to connect to a device, it might crash or halt the process. To prevent this, you can add
try
andexcept
clauses in your script. These tell Python what to do if it encounters an error when attempting to connect to a device. With proper error handling, your script becomes more resilient and able to recover gracefully from unexpected issues. - Version control with Git: It's crucial to keep track of changes when dealing with configurations. You can enhance this script by integrating it with a version control system like Git. This allows you to keep a record of all changes made to your network configurations over time. If something goes wrong, you can revert to a previous configuration, making your network more reliable and easier to manage.
Closing Up
And there you have it! A simple, yet effective way to backup your network configuration using Python. Remember, the journey is just beginning. Build on this script, enhance it, and make it your own.