In Python, a module is a file containing Python code that defines functions, classes, and variables. These modules can be reused in other Python scripts through the process of importing. Importing a module means you can access its functions, classes, and variables within your current script, allowing you to utilize external functionalities without writing them from scratch.
Imagine you're building a complex project and need to perform mathematical operations, handle date and time, or work with web data. Instead of coding all these functionalities yourself, you can import Python's built-in modules like math
, datetime
, or external libraries like requests
to easily accomplish these tasks. This not only saves time but also helps in keeping your code clean and concise.
Why do we need pip?
pip
is the package installer for Python. While Python comes with a rich standard library, there are thousands of additional packages available that extend Python's capabilities even further. These packages can be found in the Python Package Index (PyPI), a repository of software for the Python programming language. pip
helps you download and install these packages from PyPI with just a simple command. Whether you need a package for data analysis, web development, or machine learning, pip
makes it easy to add these tools to your Python environment, enhancing your programming projects with a vast array of functionalities.
Imagine Python as an iPhone. The built-in apps it comes with, like messaging or the camera, are like Python's standard library modules—ready to use for everyday tasks. Now, when you want to do something more specialized, like editing photos or managing tasks, you download new apps from the App Store. Similarly, in Python, when you need capabilities beyond the standard library, you use pip
to download external modules, akin to adding new apps to your iPhone from the App Store. This analogy highlights how Python, paired with pip
, offers both fundamental tools and the ability to expand your toolkit with specialized modules, just as iPhones do with apps.
In summary, modules and pip
significantly expand your programming possibilities in Python. By understanding how to import modules and use pip
to manage external packages, you're unlocking a treasure trove of tools that can simplify and empower your coding projects.
Python Import Example
As we discussed, to add functionality to your Python script, you can import modules using the import
statement. This allows you to use the functions and classes defined in those modules. Here is the syntax for importing modules.
import module_name
import
- import statementmodule_name
is the name of the module you want to import.
Once imported, you can access the functions and classes in the module using dot notation: module_name.function_name()
random
For our first example, we'll explore the random
module. The random
module is part of Python's standard library and provides functions that generate pseudo-random numbers for various uses, such as simulating random events, selecting random items from a list, or shuffling elements randomly. It's a versatile tool for tasks that require randomness. This module exemplifies how importing functionality can enhance your Python scripts without the need for extensive custom code.
Let's say you want to pick a random number between 1 and 10. You can use the randint
function from the random
module to do this.
import random
# Generate a random number between 1 and 10
random_number = random.randint(1, 10)
print("Random number between 1 and 10:", random_number)
- We import the entire
random
module usingimport random
. - We call the
randint
function from therandom
module, specifying the range as 1 to 10 (random.randint(1, 10)
). - The function returns a random integer within the specified range, which we then print.
datetime
Another useful module we can explore is the datetime
module. This module helps you work with dates and times in Python, offering a range of functionalities for creating, manipulating, and formatting date and time objects. Let's try and display the current date and time using the datetime
module.
import datetime
# Get the current date and time
current_datetime = datetime.datetime.now()
# Format the date and time as a string
formatted_datetime = current_datetime.strftime("%Y-%m-%d %H:%M:%S")
# Print the formatted date and time
print("Current date and time:", formatted_datetime)
#output
Current date and time: 2024-03-09 21:51:19
datetime.datetime.now()
calls thenow()
function from thedatetime
class within thedatetime
module. This function returns adatetime
object representing the current local date and time.strftime("%Y-%m-%d %H:%M:%S")
is a method called on ourdatetime
object. It formats the date and time into a string according to the specified format. In this case,%Y-%m-%d %H:%M:%S
represents the year-month-day hours:minutes:seconds.
PIP
The basic syntax for using pip
from the command line is pip install package_name
This command tells pip
to download and install the package named package_name
. After installation, you can import the package into your Python scripts using the import
statement, just like you would with any other module.
As Network Engineers, you'll find a number of external modules invaluable for automating networks. Modules like netmiko
, nornir
, and requests
provide powerful tools for managing network devices, executing remote commands, automating configurations, and interfacing with web APIs.
requests library
Let's say you need to make HTTP requests to interact with web services. One popular external module for this purpose is requests
. It simplifies the process of making HTTP requests, allowing you to interact with APIs or fetch web content with ease. To install requests
, you would use the following command.
pip install requests
import requests
# Example: GET request to fetch data from an example API
response = requests.get('https://api.example.com/data')
print(response.text)
Closing Up
There are thousands of pip modules available for various tasks and projects, but Netmiko, Napalm, Nornir, and Scrapli are among the most widely used for network automation and management. These modules provide powerful tools to interact with and automate network devices across different vendors and platforms.
In addition to these modules, there are also vendor-specific Python modules like pan-os-python
for Palo Alto Networks devices and PyEz
for Juniper's Junos OS.
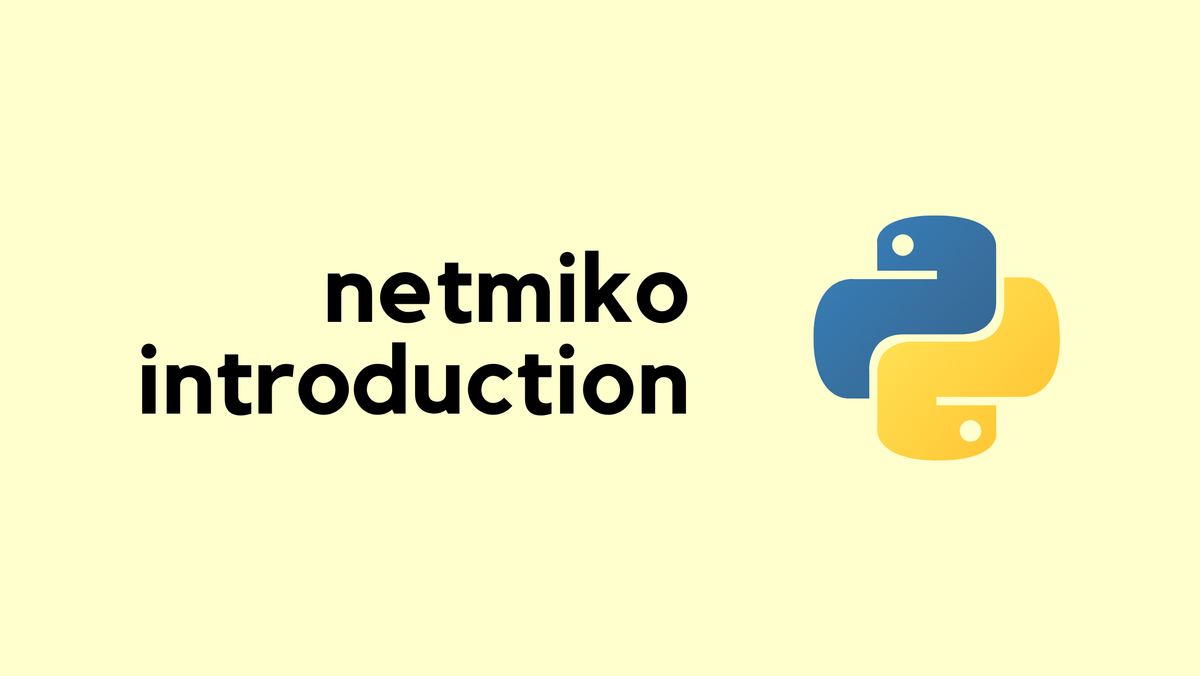