In Python, functions are a way to organize and reuse your code. They let you define a block of code that you can execute whenever you need it, simply by calling the function's name. This is incredibly useful because it helps you avoid repetition, and makes your code cleaner, and easier to understand.
Think of a function like a recipe in a cookbook. Each recipe has a name, a list of ingredients (which are like the inputs or arguments to a function), and a series of steps that tell you how to make the dish (similar to the body of the function). Just as you can follow the same recipe to make a dish multiple times, you can call a function as many times as you need, potentially with different amounts of ingredients (arguments) each time.
Python Function Syntax
The syntax for defining a function in Python is straightforward. Here's a quick overview followed by a very simple example.
def function_name(parameters):
# Block of code to execute
return value # Optional return statement
def
tells Python you're defining a function.function_name
is the name of your function. Choose something descriptive.parameters
are the inputs to your function (optional). You can define multiple parameters separated by commas.- The indented block of code under the
def
line is the body of the function, where the function's actions are defined. - The
return
statement is optional; use it if you want your function to output something.
To call a function in Python, you simply use the function's name followed by parentheses. Inside the parentheses, you include any arguments that the function expects, separated by commas if there are multiple.
function_name() #function doesn't have any arguments
function_name(argument1, argument2) #function with two arguments
A Simple Example
# Define the function
def add_two_numbers(number1, number2):
result = number1 + number2
return result
# Call the function
sum = add_two_numbers(5, 3)
print("The sum is:", sum)
#output
The sum is: 8
In this example, add_two_numbers
is a function that takes two parameters, number1
and number2
, adds them together, and returns the result. When we call add_two_numbers(5, 3)
, it computes the sum of 5 and 3, then prints "The sum is: 8" to the console. This demonstrates how functions encapsulate code for tasks you wish to perform multiple times, possibly with different input values each time.
What if we didn't use function?
Without using a function, you would need to write the code to add two numbers every time you want to perform this operation. For example, if you needed to add numbers in different parts of your program, you would repeat the addition and print statements each time.
# Adding two numbers and printing the result, repeated multiple times without a function
result1 = 5 + 3
print("The sum is:", result1)
result2 = 10 + 6
print("The sum is:", result2)
# And so on for each addition operation needed
#output
The sum is: 8
The sum is: 16
This approach can quickly make your code longer, harder to maintain, and more prone to errors, especially if the operation becomes more complex or changes over time. By using a function, you write the addition code once and simply call the function with different arguments wherever needed, making your code cleaner and more efficient.
Parameters and Arguments
Parameters and arguments are essential concepts when working with functions in Python. They allow functions to be versatile and adaptable to different inputs. Here's a closer look at how they work, followed by an example.
- Parameters are variables that you define as part of a function's definition. They represent the data that you want to pass into your function. Think of parameters as placeholders within your function for data that will be provided when the function is called.
- Arguments are the actual values or data you pass into the function when you call it. These values are assigned to the function's parameters in the order they are passed.
Let's create a function that greets a user by name. The name will be passed into the function as an argument.
# Define the function with one parameter (name)
def greet_user(name):
greeting = "Hello, " + name + "!"
return greeting
# Call the function with an argument
user_greeting = greet_user("Alice")
print(user_greeting)
#output
Hello, Alice!
- The
greet_user
function is defined with one parameter,name
, which acts as a placeholder for the name of the user we want to greet. - When calling
greet_user("Alice")
,"Alice"
is the argument that's passed to thename
parameter of the function. The function then uses this value to construct a greeting string. - The function returns the greeting, and we print it, resulting in "Hello, Alice!" being displayed on the console.
This illustrates how parameters allow a function to accept different data each time it's called, making functions more flexible and reusable.
Yet Another Example
Let's apply the concept of parameters and arguments to a simple networking-related function. We'll create a function that takes an IP address and subnet mask as arguments and returns a network configuration statement.
# Define the function with two parameters: ip_address and subnet_mask
def configure_ip(ip_address, subnet_mask):
config_statement = "Configuring IP address " + ip_address + " with subnet mask " + subnet_mask
return config_statement
# Call the function with IP address and subnet mask as arguments
network_config = configure_ip("192.168.1.10", "255.255.255.0")
print(network_config)
#output
Configuring IP address 192.168.1.10 with subnet mask 255.255.255.0
- The
configure_ip
function is designed to take two pieces of data: anip_address
and asubnet_mask
. - When we call
configure_ip
with"192.168.1.10"
and"255.255.255.0"
as arguments, these values are passed to theip_address
andsubnet_mask
parameters, respectively. - The function constructs a configuration statement using these values and returns it.
- The result, stored in
network_config
, is then printed, displaying "Configuring IP address 192.168.1.10 with subnet mask 255.255.255.0" on the console.
Python Built-in Functions
Built-in functions are pre-defined functions in Python that are readily available for use without the need for any import statements. These functions provide convenient ways to perform common tasks.
For instance, recall how we use the print()
function to display messages or results on the screen. This function is a perfect example of a built-in function. When you call print()
with a string or any other object as its argument, Python executes the function's code, which processes the argument and sends it to the console or standard output device. Here are some useful built-in functions.
len()
- Returns the length (the number of items) of an object. It can be used with strings, lists, tuples, and other data structures.print()
- Prints the specified message to the screen, or other standard output device.type()
- Returns the type of the specified object, helping you understand what kind of data you're working with.input()
- Allows the user to input a value from the terminal, which can then be used within your program.range()
- Generates a sequence of numbers, often used in loops.int()
,float()
,str()
- Convert values to an integer, floating-point number, or string, respectively.sorted()
- Returns a sorted list from the items in an iterable.max()
,min()
- Return the largest or smallest item in an iterable or two or more arguments.sum()
- Sums the items of an iterable from left to right and returns the total.
# len() - Returns the length of an object
print(len("Hello, World!")) # Output: 13
# print() - Prints objects to the terminal
print("Hello, Python!") # Output: Hello, Python!
# type() - Returns the type of an object
print(type(42)) # Output: <class 'int'>
# input() - Reads a string from standard input
# Note: Output depends on what the user types
name = input("Enter your name: ")
print(name) # Output: (Whatever the user types)
# range() - Generates a sequence of numbers
for i in range(5):
print(i) # Output: 0 1 2 3 4 (each number on a new line)
# int(), float(), str() - Convert types
print(int("10")) # Output: 10
print(float("10.5")) # Output: 10.5
print(str(10)) # Output: '10'
# sorted() - Returns a sorted list
print(sorted([3, 1, 4, 1, 5, 9, 2])) # Output: [1, 1, 2, 3, 4, 5, 9]
# max() and min() - Return the largest or smallest item
print(max(1, 3, 2)) # Output: 3
print(min('a', 'b', 'c')) # Output: 'a'
# sum() - Sums the items of an iterable
print(sum([1, 2, 3, 4, 5])) # Output: 15
Exercise - Calculate the Area of a Rectangle
Objective - Write a Python function that calculates the area of a rectangle. The function should take two parameters, the length and width of the rectangle. It should return the area.
Instructions
- Define a function named
calculate_area
that accepts two parameters.length
andwidth
. - Inside the function, calculate the area of the rectangle (Area = length * width) and return this value.
- Call your function with two arguments for length and width, and print the result to verify that your function works correctly.
Hints
- Remember to use the
return
statement to give back the area from your function. - You can test your function with different values for length and width to see if it calculates the area correctly.
Once you've written your function, try calling it with some sample values like calculate_area(5, 3)
and see if it prints the expected result, which should be 15
for this specific call. This exercise will help solidify your understanding of how functions use parameters to accept inputs and how they can return a result.
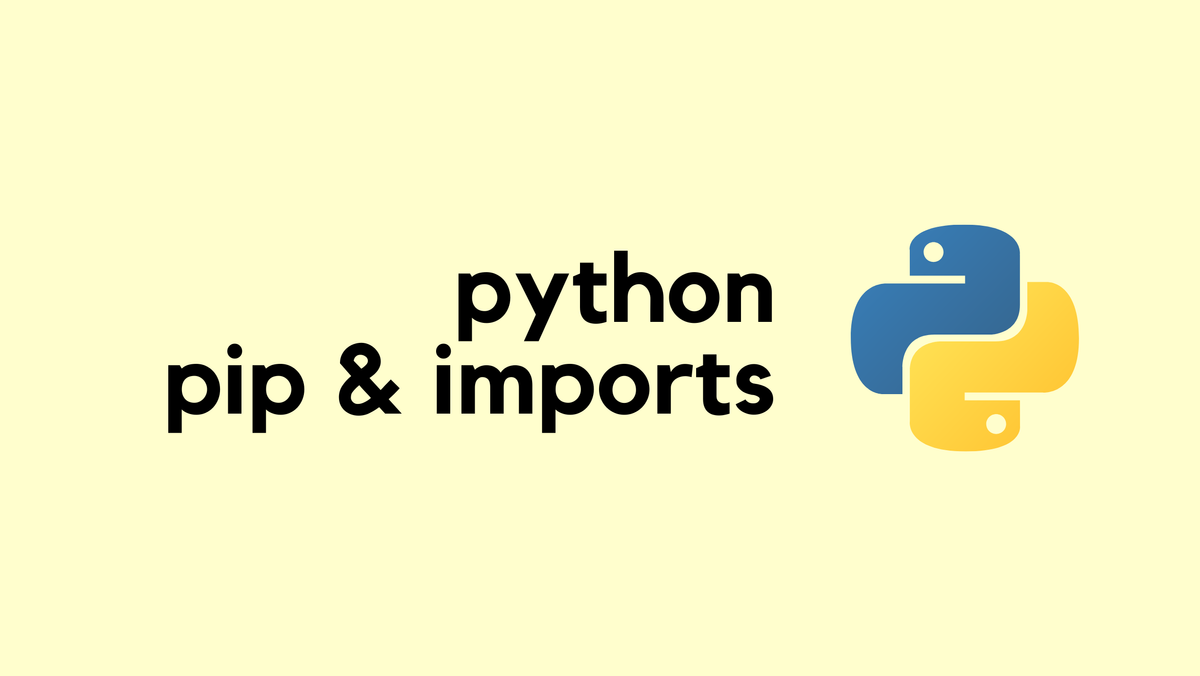