In programming, flow control is all about making decisions in your code. It's how you can tell your program to run different pieces of code based on certain conditions or repeat some action multiple times. This ability to control the flow of execution is fundamental, as it allows your programs to respond to different inputs and situations dynamically.
if, elif and else
Python, like other programming languages, provides several constructs for flow control, including if
, elif
, and else
statements for conditional execution, as well as loops for repeating actions. In this section, we're going to focus on conditional statements.
Conditional statements allow your program to execute certain blocks of code depending on whether a condition is true or not. This concept is akin to making decisions in real life based on the information you have. For example, if it's raining, you might decide to take an umbrella. In a Python program, you can write a similar condition to decide what action to take based on the data you're working with.
Let's break down the syntax and logic of if
, elif
, and else
statements in Python. These statements allow your program to evaluate conditions and execute specific blocks of code depending on the outcome of these evaluations.
'if' Statement
The if
statement is the most basic form of conditional execution in Python. It checks a condition, and if that condition evaluates to True
, it executes the block of code indented under it.
if condition:
# Block of code executes if the condition is True
- condition - This is an expression that evaluates to either
True
orFalse
. - If the condition is
True
, the code block under theif
statement runs. - If the condition is
False
, the code block is skipped.
'elif' Statement
elif
is short for "else if". It allows you to check multiple conditions after an if
statement. If the condition for the if
statement is False
, it moves on to the elif
condition, checks it, and if it's True
, executes the block of code under it.
if condition1:
# Executes this block if condition1 is True
elif condition2:
# Executes this block if condition1 is False and condition2 is True
- You can have multiple
elif
statements following anif
, allowing you to check a series of conditions. - Each
elif
is checked in order. Once anelif
condition evaluates toTrue
, its block executes, and the rest of theelif
blocks are skipped.
'else' Statement
The else
statement catches anything which isn't caught by the preceding if
and elif
conditions. It does not have a condition; instead, it executes its block of code if all the previous conditions are False
.
if condition1:
# Executes this block if condition1 is True
elif condition2:
# Executes this block if condition1 is False and condition2 is True
else:
# Executes this block if all the above conditions are False
The else
block is optional. However, including it can be useful for handling unexpected cases or providing a default action.
Logical Flow
The logical flow of these statements works like this.
- The
if
condition is checked first. If it'sTrue
, its block executes, and the program skips anyelif
orelse
blocks. - If the
if
condition isFalse
, the program checks theelif
conditions in the order they appear. The firstelif
condition that evaluates toTrue
has its block executed, and then the program exits the conditional structure. - If none of the
if
orelif
conditions areTrue
, and anelse
block exists, theelse
block executes.
This structure allows you to branch your code into multiple paths and make decisions on which path to follow based on the conditions you specify.
Simple Example
Let's use a simple example that involves checking the status of a network device. Imagine you have a variable that stores the operational status of a router. Based on this status, you want to print a message that indicates whether the router is up, down, or in maintenance mode.
# Variable storing the router's operational status
router_status = "up"
# Conditional checks using if, elif, and else
if router_status == "up":
print("The router is operational")
elif router_status == "down":
print("The router is down")
elif router_status == "maintenance":
print("maintenance mode")
else:
print("Unknown router status")
- If the
status
is"up"
, it prints that the router is operational. - If the
status
changes to"down"
, it suggests checking connections or configurations. - If the
status
is"maintenance"
, it indicates that the router is under maintenance. - The
else
part covers any status that is not recognized, printing an unknown status message.
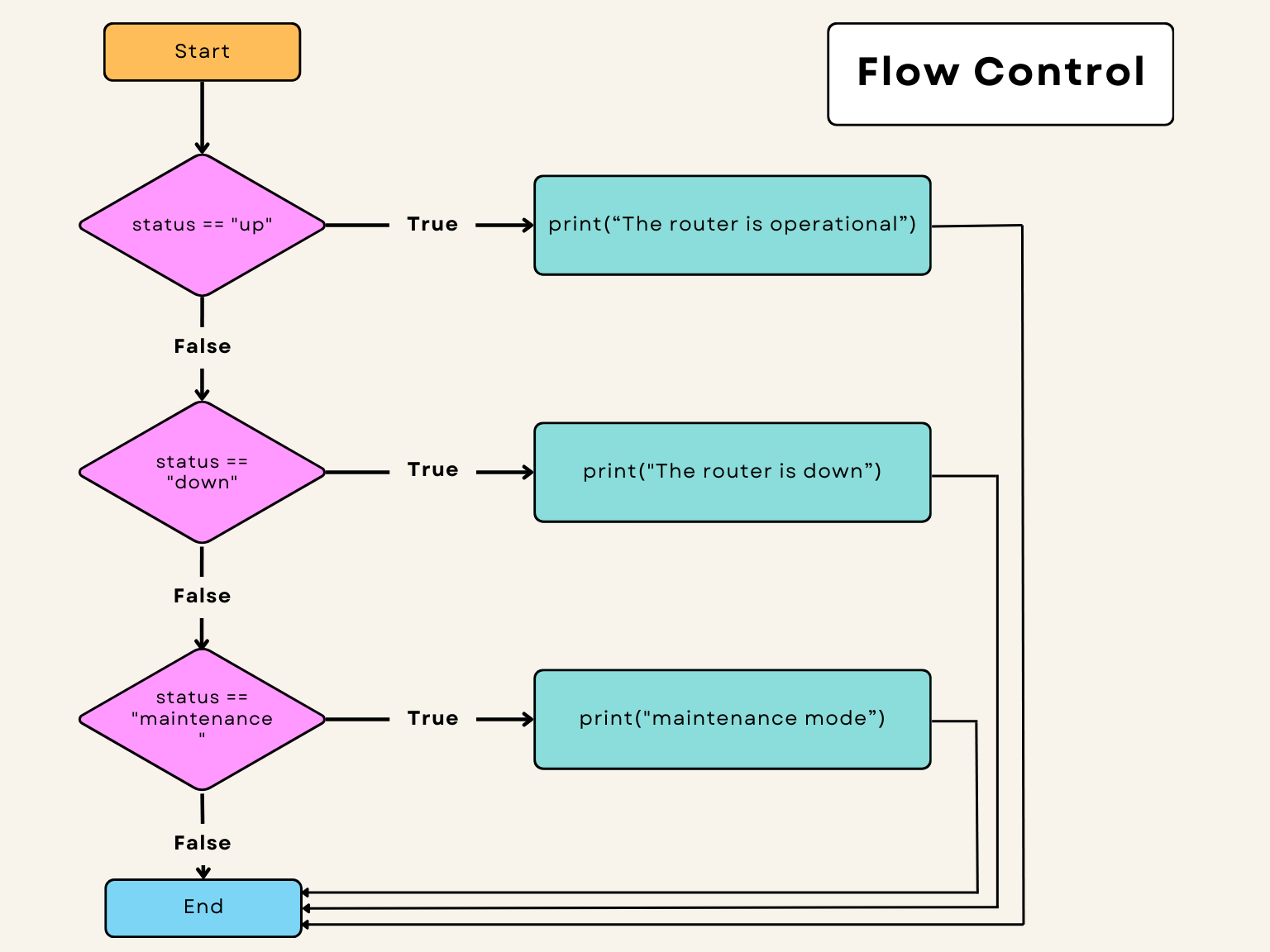
Conditional Exercise
Objective - Write a Python script that asks the user to enter the current hour (in 24-hour format) and then prints a personalized greeting based on the time of day.
- Prompt the user to input the current hour (e.g., 9, 14, 23) as an integer.
- Based on the input, print a greeting as follows.
- "Good morning!" if the hour is between 5 and 11 (inclusive).
- "Good afternoon!" if the hour is between 12 and 17 (inclusive).
- "Good evening!" if the hour is between 18 and 21 (inclusive).
- "Good night!" if the hour is between 22 and 4 (inclusive).
- If the user enters an invalid hour (less than 0 or greater than 23), print "Invalid input."
Here is the answer but before you look at it, please try it by yourself 😄
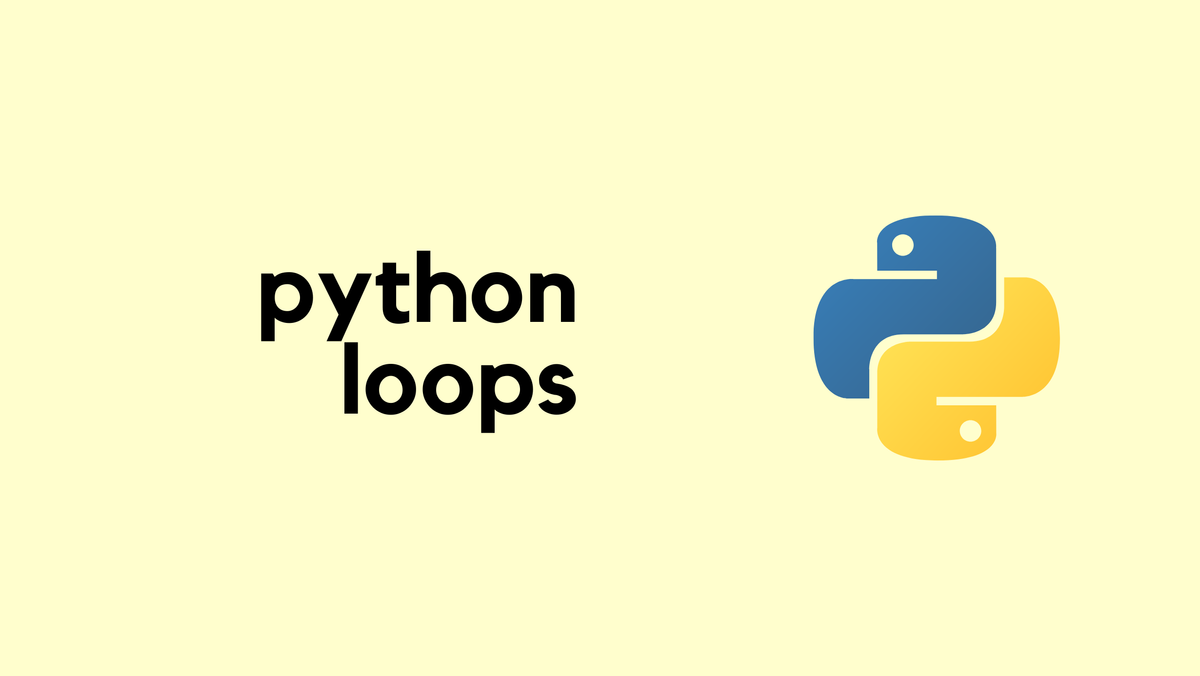