In programming, loops are powerful tools that allow you to repeat a block of code multiple times. This capability is crucial because it saves you from having to write the same code over and over again for tasks that need to be repeated. Instead, you can use loops to automate and manage these repetitions efficiently.
Why do we need loops?
Why do we need loops? Imagine you're tasked with performing an action multiple times, such as checking the status of different network devices, processing multiple user inputs, or iterating through a list of IP addresses. Writing separate lines of code for each repetition is not practical, especially as the number of repetitions grows. Loops solve this problem by allowing you to execute a block of code as many times as necessary, based on the conditions you set.
'While' and 'For' Loops
In this part of the course, we'll cover two main types of loops in Python, while
loops and for
loops.
While
loops execute as long as a specified condition is True. They're useful when you want to repeat an action but the exact number of repetitions isn't known in advance.For
loops are used for iterating over a sequence (such as a list, tuple, dictionary, or string) and executing a block of code for each item in the sequence. They're ideal when you know the number of repetitions or want to perform an action on each item in a collection.
We'll also touch on the break
statement, which provides you with the ability to stop the loop before it has looped through all the items.
Lastly, we'll explore the range()
function, a handy tool for generating sequences of numbers, often used in loops to specify the number of times the loop will run.
'While' loops
A while
loop in Python repeatedly executes a block of code as long as a given condition is True. It's a powerful tool for when you need to perform an action multiple times, but the number of iterations needed isn't known before the loop starts. This type of loop keeps running until the condition becomes False or the loop is explicitly terminated.
while condition:
# Block of code to execute
condition - This is an expression that the loop evaluates before each iteration. If the condition is true, the loop continues with the next iteration. If it's false, the loop ends.
While Loop Example - Countdown Timer
Let's say you want to create a simple countdown timer that prints numbers starting from a specified number down to 1. A while
loop is perfect for this scenario because it can keep decreasing the number and stop when it reaches 1.
# Starting number for the countdown
countdown = 5
# While loop for the countdown
while countdown > 0:
print(countdown)
countdown -= 1 # Decrease the countdown by 1 in each iteration
print("Blast off!")
#output
5
4
3
2
1
Blast off!
In this example, the while
loop checks if the countdown
variable is greater than 0. If true, it prints the current value of countdown
and then decreases countdown
by 1. This repeats until countdown
is no longer greater than 0, at which point the loop ends, and "Blast off!" is printed.
This simple countdown timer illustrates how a while
loop can perform repetitive actions with a clear condition for ending the loop. The key is to ensure the condition will eventually become false; otherwise, you might create an infinite loop that never ends.
'For' loops
A for
loop in Python is used to iterate over a sequence (such as a list, tuple, dictionary, string) or any other iterable object and execute a block of code for each item in the sequence. Unlike while
loops, which continue until a condition becomes false, for
loops are typically used when the number of iterations is known or defined by the iterable's length. This makes for
loops especially useful for going through data structures item by item, performing operations, or generating sequences of numbers. Here is the syntax.
for item in sequence:
# Block of code to execute
- sequence - This is the iterable object (list, tuple, string, etc.) you want to iterate over.
- item - On each iteration of the loop,
item
takes the value of the next item in the sequence, and the block of code is executed.
for
loop in Python, the variable name that temporarily holds each value in the sequence can be anything you choose; it doesn't have to be item
. This name is simply a placeholder that represents the current element of the sequence as the loop iterates through it. It's best to choose a meaningful name that makes your code easy to read and understand.For Loop Example - Grocery List
Imagine you have a grocery list and you want to print each item on the list. A for
loop is perfect for this scenario because it allows you to iterate over the list and perform an action for each item, in this case, printing the item's name.
# List of grocery items
grocery_list = ['apples', 'bananas', 'carrots', 'bread']
# For loop to print each grocery item
for item in grocery_list:
print("Need to buy:", item)
In this example, the for
loop goes through each item in grocery_list
. With each iteration, it assigns the current item to the variable item
and then executes the block of code indented under the for
statement, which prints out the item. This will result in each grocery item being printed on its own line:
#output
Need to buy: apples
Need to buy: bananas
Need to buy: carrots
Need to buy: bread
For Loop Example - Check Device Status
Imagine you have a list of network devices and their statuses. You want to check each device and print a message if a device is offline.
# List of device statuses
devices = ['online', 'offline', 'online', 'offline', 'online']
# For loop to check each device
for status in devices:
if status == 'offline':
print("A device is offline!")
else:
print("A device is online")
#output
A device is online
A device is offline!
A device is online
A device is offline!
A device is online
In this example, the for
loop iterates over the devices
list. For each iteration, it checks the status
of a device. If a device's status
is 'offline'
, it prints "A device is offline!". Otherwise, it prints "A device is online." This way, you can quickly assess the status of each device in your network.
For
loops are incredibly versatile and can be used with a wide range of data types, making them indispensable for data processing and iteration in Python.
Break statement
The break
statement in Python is used to exit a loop prematurely, even if the loop's condition has not become False. It provides a way to "break" out of the loop from within its body, typically under a specific condition that you define. This can be particularly useful when searching for an item in a collection or when you want to stop the loop once a certain condition is met, without having to wait for the loop to iterate through all items.
Imagine you have a list of device statuses, and you want to check if any device is offline. Once you find the first offline device, there's no need to continue checking the rest.
device_statuses = ['online', 'online', 'offline', 'online', 'offline']
for status in device_statuses:
if status == 'offline':
print("Found an offline device. Stopping search.")
break # Exit the loop immediately
In this example, the for
loop iterates through each item in device_statuses
. When it encounters a device with the status 'offline'
, it prints a message and then executes the break
statement. The break
causes the loop to end immediately, and no further items in the list are checked. This is efficient because it stops the loop as soon as it finds what it's looking for, saving time and resources.
range() functions
The range()
function in Python generates a sequence of numbers. It is commonly used in loops to specify how many times the loop should run. The range()
function can take one, two, or three arguments, start (optional, default is 0), stop (required), and step (optional, default is 1).
Syntax of range()
range(stop)
- Generates numbers from 0 up to, but not including,stop
.range(start, stop)
- Generates numbers fromstart
up to, but not including,stop
.range(start, stop, step)
- Generates numbers fromstart
up to, but not including,stop
, incrementing bystep
.
Let's use the range()
function with its simplest form, range(stop)
, to print the first 5 integers.
for i in range(5):
print(i)
#output
0
1
2
3
4
range(5)
, it starts from 0
and not 1
because Python, like many other programming languages, uses zero-based indexing. This means that the first item in a sequence is indexed at 0
rather than 1
. This approach is based on the way memory is accessed in low-level programming, where an offset of 0
signifies the starting point. Therefore, when you use range(5)
, it generates a sequence from 0
to 4
, aligning with Python's indexing system. For Loop Exercise - Sum of Positive Numbers
Objective - Write a Python script that calculates the sum of all positive numbers in a given list of numbers.
- Here's a list of numbers -
numbers = [1, -2, 3, 4, -5, 6, -7, 8]
- Use a
for
loop to iterate through each number in the list. - If a number is positive, add it to a sum variable.
- After the loop completes, print the total sum of the positive numbers.
Hints
- Initialize a variable
total_sum
to0
before starting the loop. - Use an
if
statement within the loop to check if the current number is greater than 0. - Don't forget to print
total_sum
after the loop to see the result.
Expected Output - The program should output the sum of all positive numbers in the list, which is 22
for the provided list.
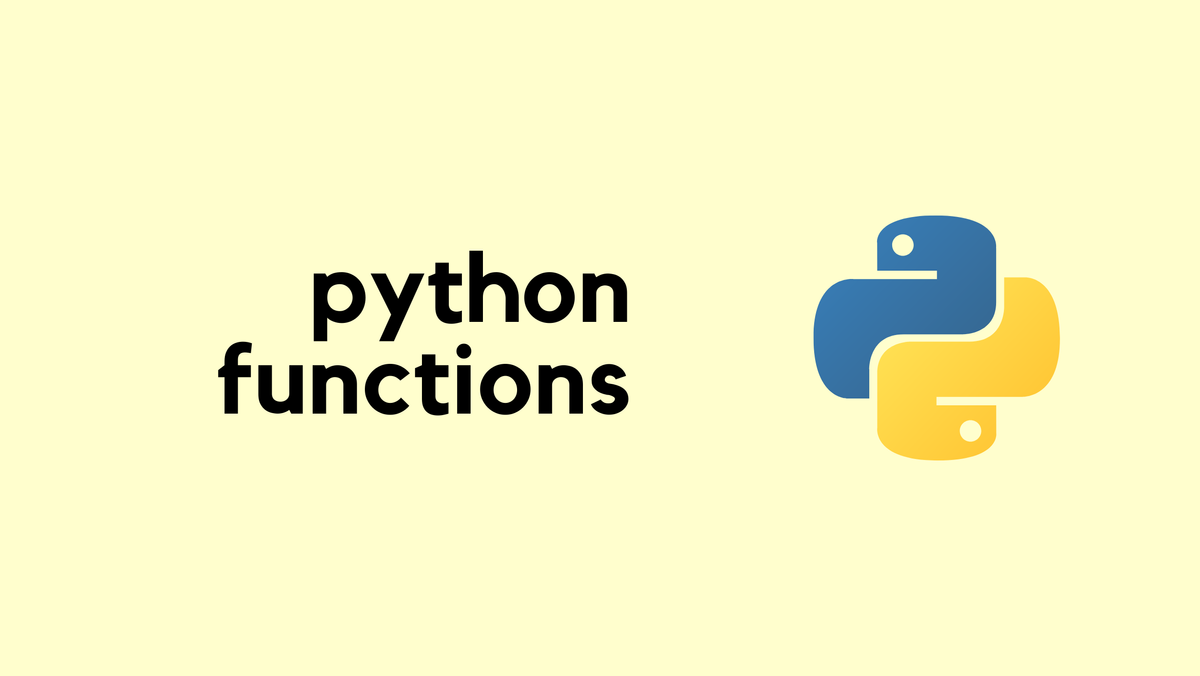