In this second part, we will cover how to install Python and VS Code and then run a very basic program. Our ultimate goal here is, when we run this Python code, we want the code to print out Hello World!
in our terminal. If you can do this successfully, welcome to the Python Club.
Python Installation
If you're looking to install Python, there are many helpful videos and guides for different operating systems. If you're on Windows or Mac and need guidance, there are plenty of good articles and resources online that can help you through the installation process step by step.
Windows - https://www.digitalocean.com/community/tutorials/install-python-windows-10
As for me, I'm using Linux, specifically Ubuntu, so I've shared how you can install Python on Ubuntu.
root@b70e1a198a7d:/# apt update
root@b70e1a198a7d:/# apt install python3
Reading package lists... Done
Building dependency tree... Done
Reading state information... Done
The following additional packages will be installed:
libexpat1 libmpdec3 libpython3-stdlib libpython3.10-minimal libpython3.10-stdlib libreadline8 libsqlite3-0 media-types python3-minimal
python3.10 python3.10-minimal readline-common
Suggested packages:
running python post-rtupdate hooks for python3.10...
Processing triggers for libc-bin (2.35-0ubuntu3.6) ...
root@b70e1a198a7d:/# python3
Python 3.10.12 (main, Nov 20 2023, 15:14:05) [GCC 11.4.0] on linux
Type "help", "copyright", "credits" or "license" for more information.
root@b70e1a198a7d:/# python3 --version
Python 3.10.12
python vs python3
On macOS and Linux systems, you might encounter two different terminal commands for running Python: python
and python3
. This distinction exists mainly due to the transition from Python 2 to Python 3, which are incompatible with each other in some significant ways.
python
usually refers to Python 2 on older systems. For a long time, Python 2 was the default version of Python installed on many Linux distributions and macOS.python3
explicitly refers to Python 3, the current and actively developed version of Python. It includes new features and improvements over Python 2, making it the preferred choice for new projects.
The reason for having these two commands is to allow both Python 2 and Python 3 to coexist on the same system without conflict, catering to applications and scripts that rely on a specific version. Over time, as Python 2 has reached the end of its life (EOL in January 2020), python3
is becoming the standard command to invoke Python, and the python
command is increasingly being linked to Python 3 in newer system installations and environments.
python --version
doesn't point to Python 3, try using python3 --version
to locate it. Moving forward, use the command that points to Python 3.x for your work. This small step ensures that you're using the most current and supported version of Python, keeping your projects up-to-date and secure.Visual Studio Code
Now that we've got Python set up, our next step is to install VS Code. Why VS Code? It's a powerful editor that's not just easy to use but also packed with features that make coding simpler, like syntax highlighting, code completion, and debugging tools. It's a favourite among many developers for these reasons.
After installing VS Code, go ahead and create a new directory (or folder) anywhere you like. This will be our workspace or project folder. Open this directory in VS Code to start creating and managing your files directly from the editor.
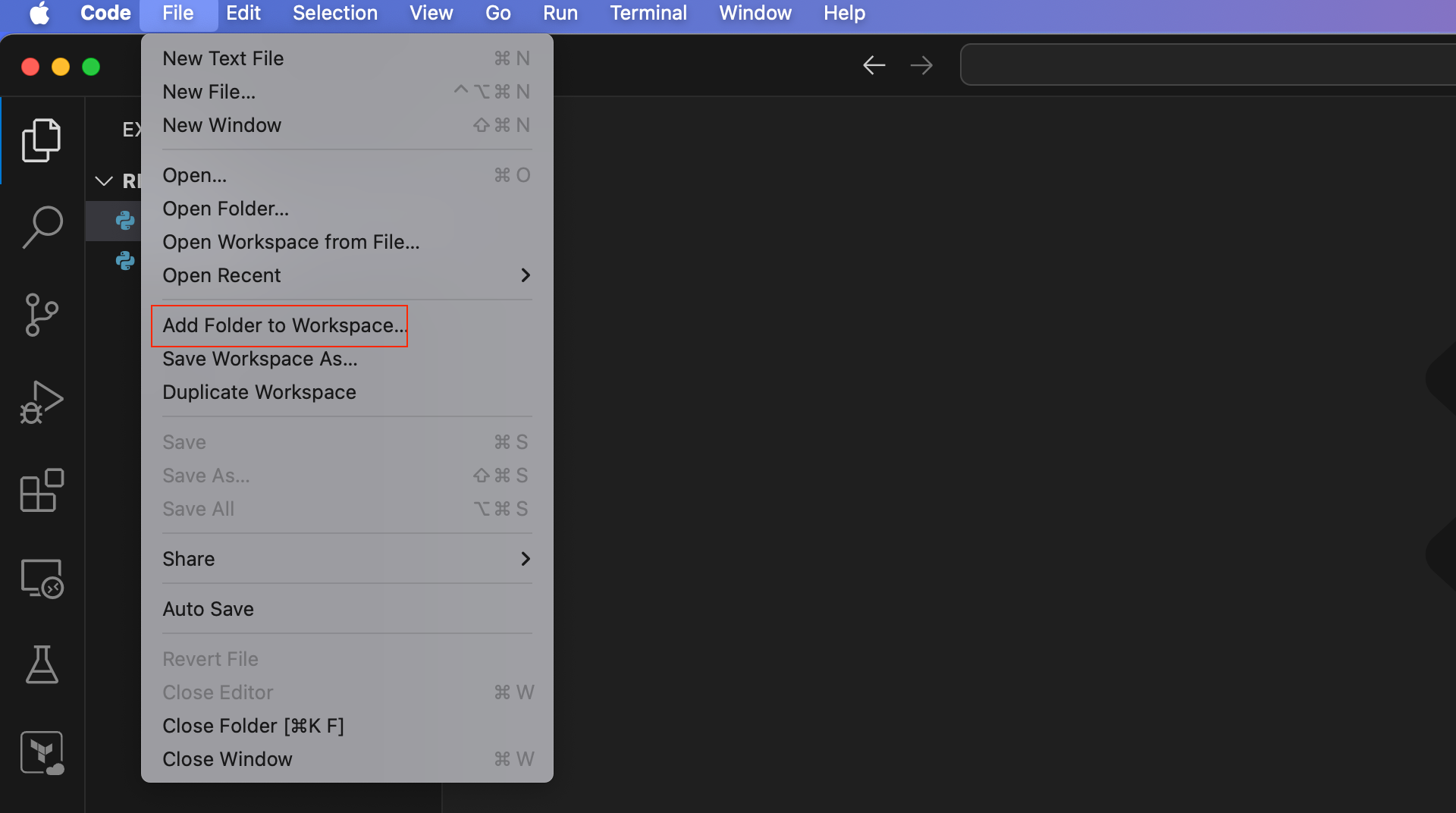
VS Code also has an integrated terminal, so you can run commands or scripts without leaving the editor. To access it, go to Terminal > New Terminal
. This convenience means you won't have to switch back and forth between VS Code and your system's terminal, streamlining your workflow.
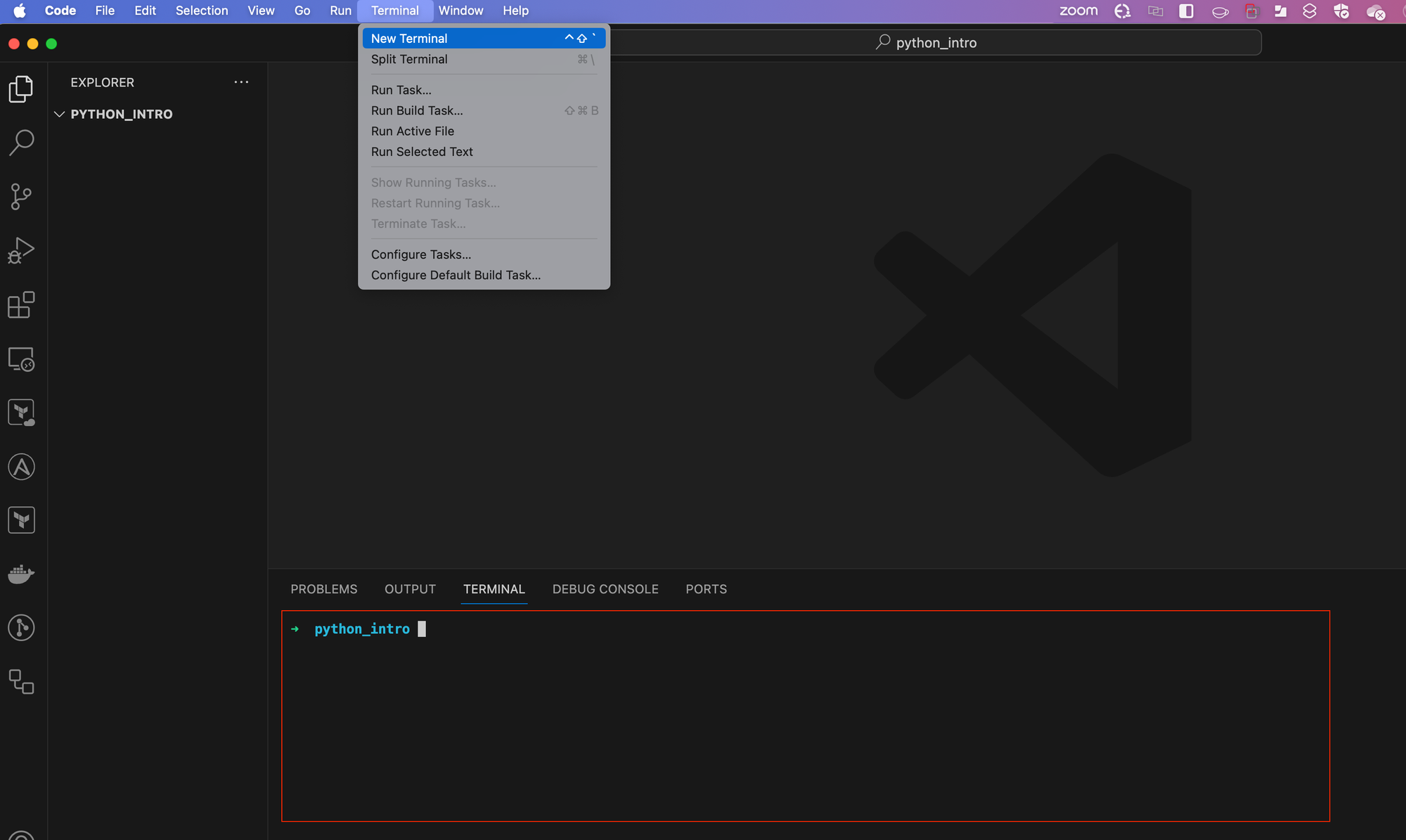
Our First Code
To get started with our first Python program, we need to create a Python file. You can name this file anything you like, but for our example, let's call it my_first_script.py
. This naming helps us remember that this is our very first step into Python programming.
Inside my_first_script.py
, we're going to add a single line of code.
#my_first_script.py
print('Hello World!')
When we run this file (by running python my_first_script.py
on the terminal), the goal is for our terminal to display the message: 'Hello World!'. Let's break down how this works and what each part of the line means.
print
- This is a built-in Python function that outputs information to the terminal. Think of it as a way of sending messages from your code to the screen, so you can see what your program is doing or thinking.('Hello World!')
- These are the contents we want to print. In Python, anything enclosed in quotes (single'
or double"
quotes) is considered a string, which is a type of data representing text.- The whole line
print('Hello World!')
- When Python executes this line, it calls theprint
function and passes 'Hello World!' as an argument. The function then does its job by displaying 'Hello World!' in your terminal.
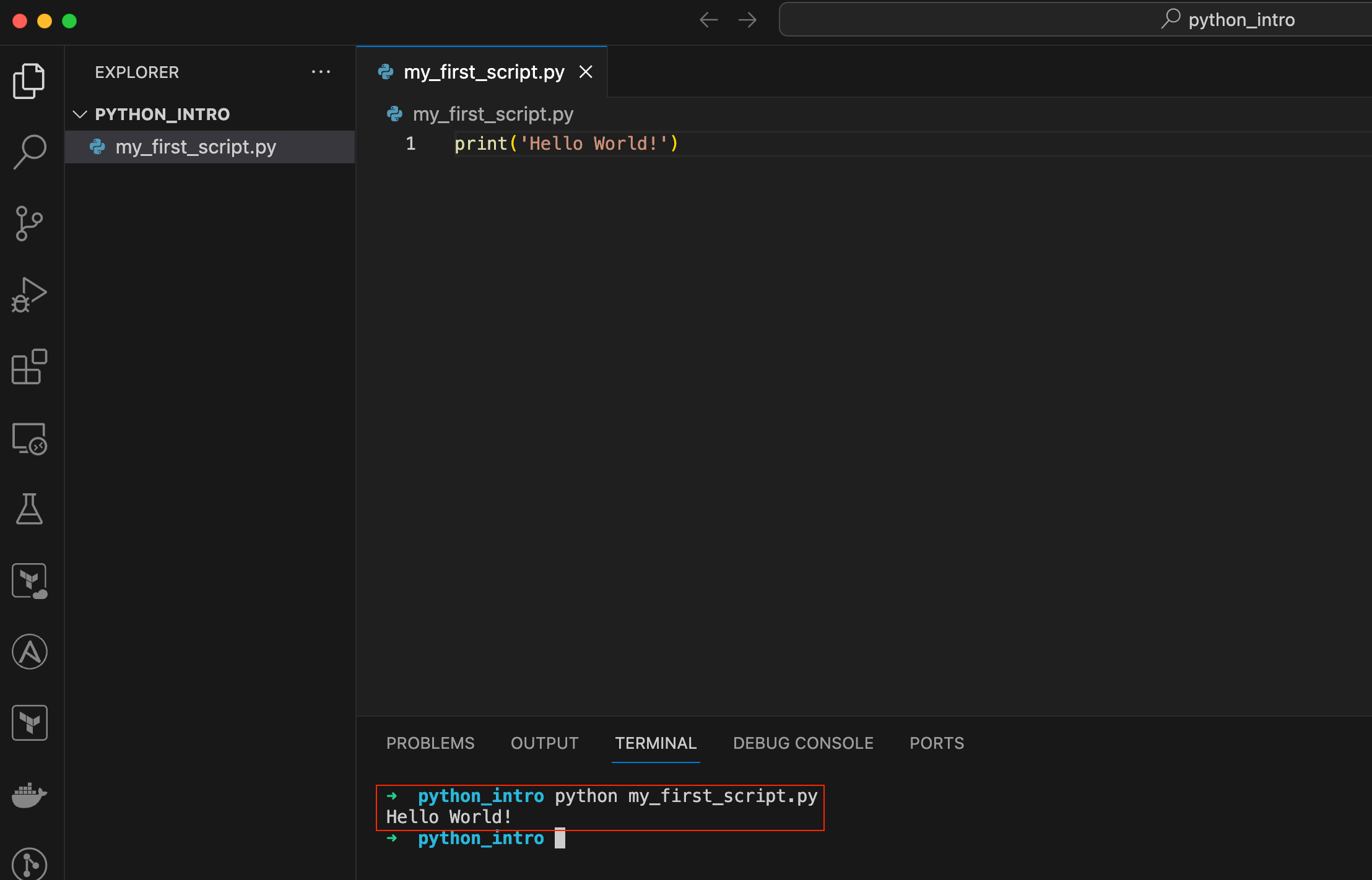
By running this simple line of code, you're telling Python to execute a command that outputs a message. This is a fundamental concept in programming, using functions to perform tasks, and in this case, the task is to display a message.
To run this command, just open the terminal in your VS Code and type python my_first_script.py
as shown in the screenshot. This is how you'll make your Python script work and see what it does. Running your script from the VS Code terminal is simple and keeps everything in one place. When you see 'Hello World!' on your screen, you've taken your first step into Python programming, showing that you've written and run your first Python script successfully.
Multiple Print Statements
Another useful thing to know about the print
function is that you can use it multiple times to display different pieces of information on separate lines. This is very handy when you want to share several messages or pieces of data one after the other. For example, if you want to print a greeting and then ask a question, you could write.
print('Hello World!')
print('How are you today?')
#output
Hello World!
How are you today?
Each print
statement works independently, so each message appears on its own line. This way, you can organize your output to make it clear and easy to read.
Feel free to try adding more print
statements to your script to see how they work together. It's a great way to get comfortable with writing and running Python code.
Some Tips
For this part of the course, focusing on the 'Hello World!' program and the print
function, there's one more thing you might find helpful. The print
function is one of the most basic yet powerful tools in Python. It's not just for saying 'Hello World!'—you can use it to display any kind of information you want, from error messages to the results of complex calculations.
Here's what you should remember.
- You can print multiple items at once by separating them with commas. For example,
print("The answer is", 42)
would output,The answer is 42
. - You can also use the
print
function to display the values of variables. If you have a variablea
with the value 10, writingprint(a)
will show10
on your screen. (We will cover this in detail later)
Exercise - Personalized Greeting Program
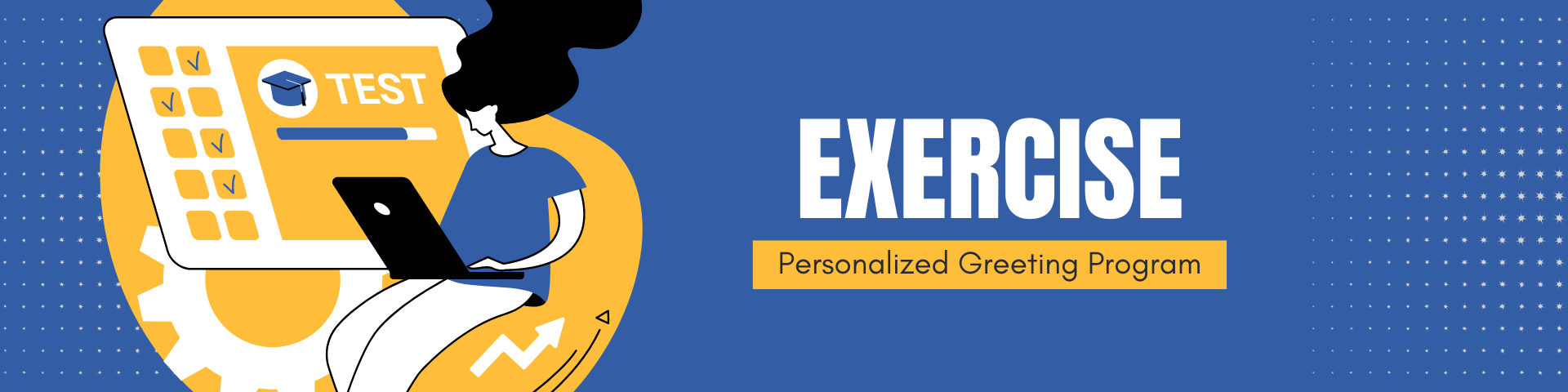
Get the User's Name - First, your script should ask for the user's name. You can achieve this by using the input
function like so.
name = input('What is your name? ')
This line of code will display the message What is your name?
and wait for the user to type their name and press Enter. Whatever they type gets stored in the variable name
.
Print a Personalized Greeting - Next, use the print
function to display a personalized greeting using the name the user entered. Try to use an f-string to include the variable in your message, like this.
print(f'Hello, {name}! Welcome to the Python Club.')
Expected Output - The program should ask for the user's name and then greet them by name. For example, if the user types in "Alex", the output should be.
What is your name? Alex
Hello, Alex! Welcome to the Python Club.
input
function and f-strings
. If you don't understand them right away, don't worry. We will cover them in more detail later in the course.F-strings, introduced in Python 3.6, offer a more readable and concise way to format strings. They are called "f-strings" because you prefix the string with the letter "f" before your opening quotation mark. This tells Python to consider the string as an f-string and to evaluate any expressions within curly braces {}
directly.
{name}
within the f-string is a placeholder that gets replaced by the value of the variable name
. When Python executes this code, it evaluates the expression inside the curly braces and inserts the result into the string. So, if name
is "Alex"
, the output will be: "Hello, Alice! Welcome to the Python Club."
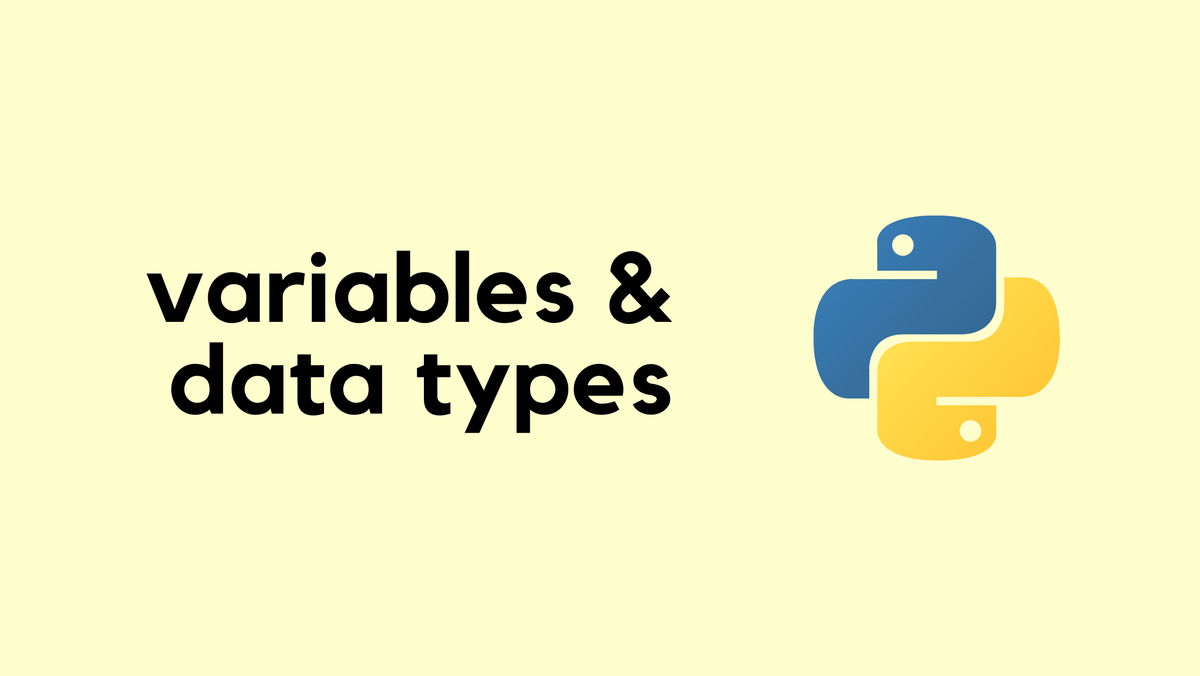