In this part of the course, we'll dive into variables and data types, two fundamental concepts in Python programming. Variables are like containers that store information you can use and manipulate in your code, while data types describe the kind of data a variable holds, such as text, numbers, or true/false values. Understanding these concepts is crucial for writing any Python program, as they form the building blocks of your code. We'll explore how to create variables, assign values to them, and use different data types to handle various kinds of information. Keep it simple, and you'll see how these concepts apply in real-world programming scenarios.
Variables
In Python, a variable is like a container or a label that can store information or data. Think of it like a box that you can put different things in, such as numbers, words, or even a list of things. In Python, you can create a variable by giving it a name and then assigning a value to it using the equal sign =
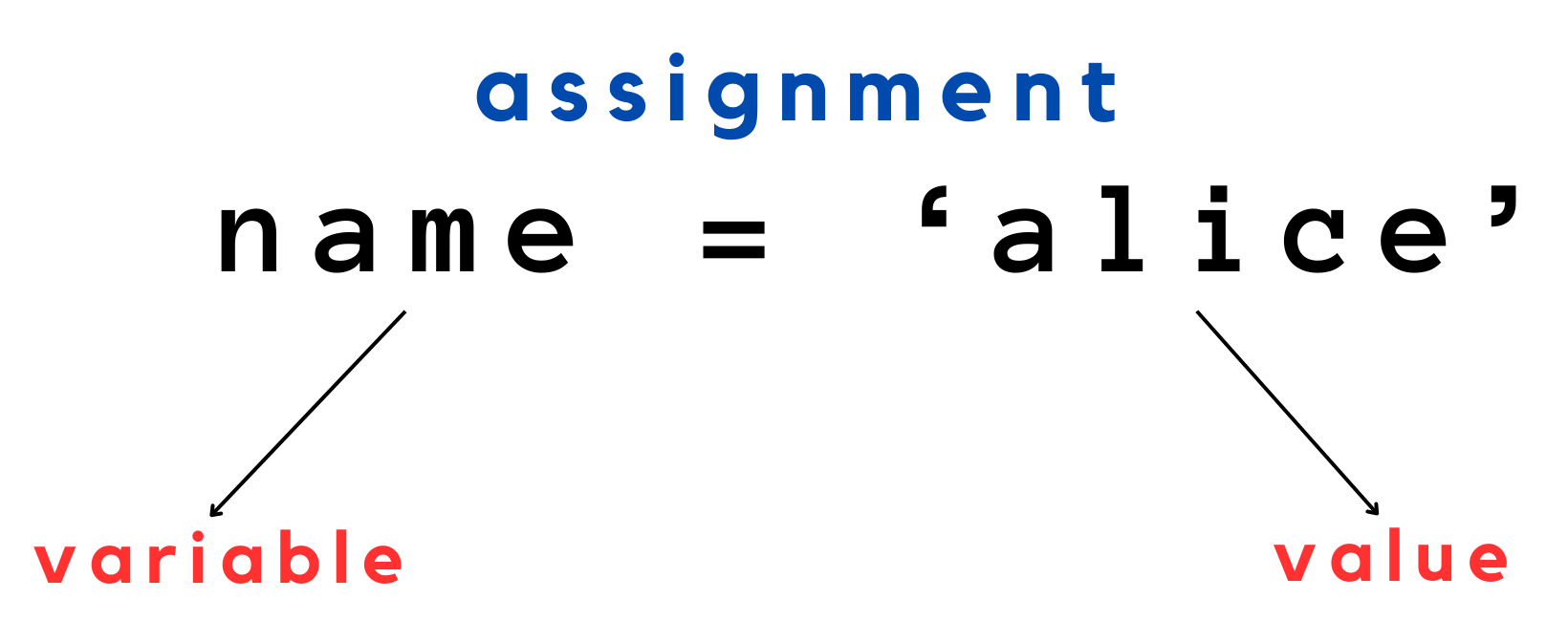
Variables are used to store data in a program. In Python, you don't need to declare the type of a variable. Instead, Python determines the type based on the value assigned to the variable. Here's an example:
# Assigning a string to a variable
name = "alice"
# Assigning an integer to a variable
x = 10
# Assigning a float to a variable
pi = 3.14
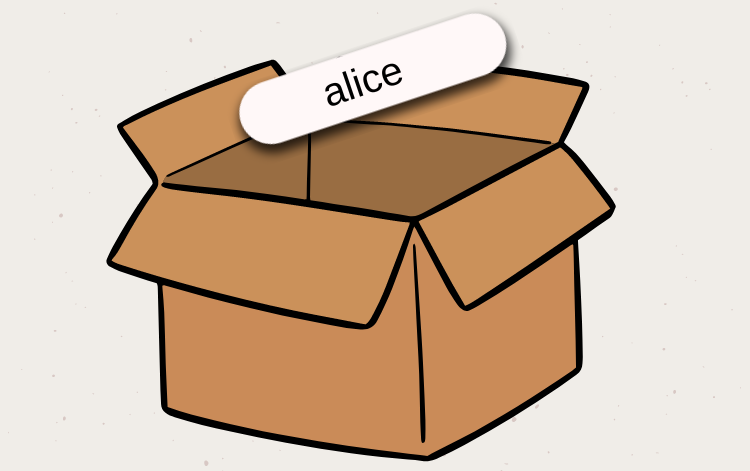
Now, whenever you use name
in your code, Python will understand that you're referring to the value alice
. You can use these variables in various operations, like adding numbers or combining text.
name
for a variable that stores a person's name or pi
for a variable that stores the value of π makes your code more readable not just to others, but also to you, especially if you come back to it after some time.Introduction to Data Types
When we start programming in Python, one of the first things we need to understand is the concept of data types. But what exactly are data types, and why do they matter? In simple terms, data types tell Python (or any programming language, for that matter) what kind of data we're working with. This could be anything from numbers and text to more complex types of data like lists of items or collections of key-value pairs.
Why is this important? Because the data type of a piece of information affects what kinds of operations we can perform on it. For example, we can add and subtract numbers, but trying to do the same with text wouldn't make much sense. Similarly, some operations are specific to text, like joining two pieces together or capitalizing them.
Python is known for being flexible and easy to work with, partly because it automatically recognizes the type of data you're using. However, as a programmer, understanding these types and how they work is crucial. It helps you make better decisions about how to store, manipulate, and use your data effectively in your programs.
In the following sections, we'll explore the main data types available in Python. These include numeric types like integers and floating-point numbers, text in the form of strings, collections like lists, tuples, and dictionaries, and the boolean type, which represents true and false values. Each of these data types has its unique characteristics and uses, which we'll dive into next.
Basic Data Types
In the Basic Data Types section, we're going to explore the core elements that form the foundation of Python programming. These types are the simplest forms of data you can work with and are essential for performing a wide range of programming tasks. Here's what we'll cover.
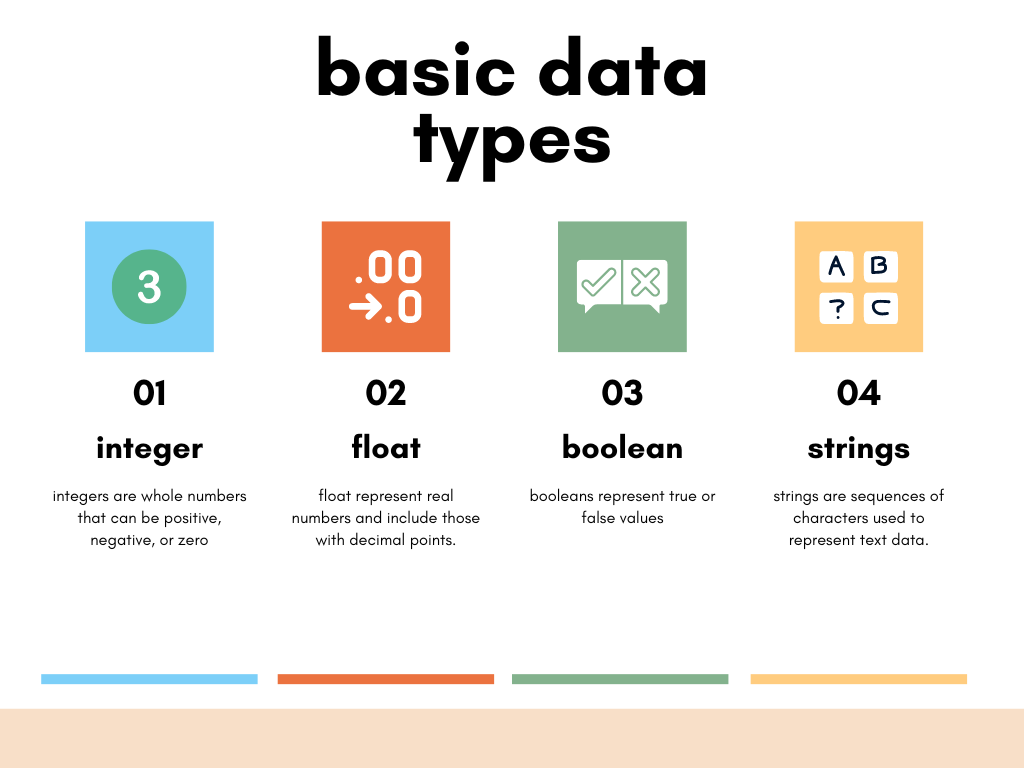
- Integers - We'll start with integers, which are whole numbers that can be positive, negative, or zero.
- Floats - Next, we'll dive into floats, which represent real numbers and include those with decimal points.
- Booleans - We'll also cover Booleans, a simple yet powerful type representing true or false values. Booleans are fundamental in making decisions in your code, allowing you to execute different actions based on certain conditions.
- Strings - Finally, we'll explore strings, which are sequences of characters used to represent text data. Strings are incredibly versatile and can be used for anything from displaying messages to users, to processing text data in your applications. (As we've seen in the previous part of printing text/string to the terminal)
Integer
In Python, an integer is a whole number that can be either positive, negative, or zero. It's one of the most common data types used in programming for counting, indexing, and performing various arithmetic operations.
age = 25
temperature = -5
Integers are perfect for situations where you need to keep track of quantities that don't have fractional parts, like the number of users registered on a website or the temperature in degrees Celsius on a cold day.
Floats
Floats, or floating-point numbers, represent real numbers and can contain decimal points. They are crucial when you need more precision in calculations than integers can provide.
price = 19.99
average_score = 82.5
Floats are used when dealing with measurements, such as calculating the price of an item after-tax or averaging scores in a game where fractions can occur.
Booleans
The Boolean data type has only two possible values: True
or False
. Booleans are extremely useful in controlling the flow of a program with conditional statements.
is_raining = False
is_authenticated = True
Booleans are ideal for checking conditions, such as whether it's raining before deciding to take an umbrella, or verifying if a user is authenticated before allowing access to a secure part of a website.
Strings
Strings are sequences of characters used to represent text. They are enclosed in quotes, and you can use either single ('
) or double ("
) quotes.
greeting = "Hello, World!"
name = 'Bob'
Strings are used in nearly every aspect of programming that involves text, from displaying messages to users and collecting input like names and addresses, to working with files and data.
Collection Data Types
Collection data types in Python are used to store multiple items in a single variable. They are particularly useful when you need to work with, manipulate, or organize large amounts of data. Python provides four built-in collection data types that are listed below.
We'll spend more time on lists and dictionaries because you'll use them a lot in Python. They help you organize your data well. I don't use sets and tuples as much, but lists and dictionaries are key for most things you'll do.
List
Lists are ordered and mutable collections that can hold items of different data types. They are denoted by square brackets []
. In a networking context, you might use a list to store a series of IP addresses or device names.
ip_addresses = ['192.168.1.1', '10.0.0.1', '172.16.0.1']
device_names = ['Router1', 'Switch1', 'Firewall1']
Lists are described as "ordered" and "mutable" collections. Being "ordered" means that the items in a list appear in a specific sequence — the order in which you add items is the order they'll stay in, and you can use their position or index to access them. "Mutable" means that you can change a list after creating it; you can add, remove, or alter items. These characteristics make lists versatile and widely used in Python programming.
Accessing Items
vendors = ['cisco', 'juniper', 'nokia', 'arista']
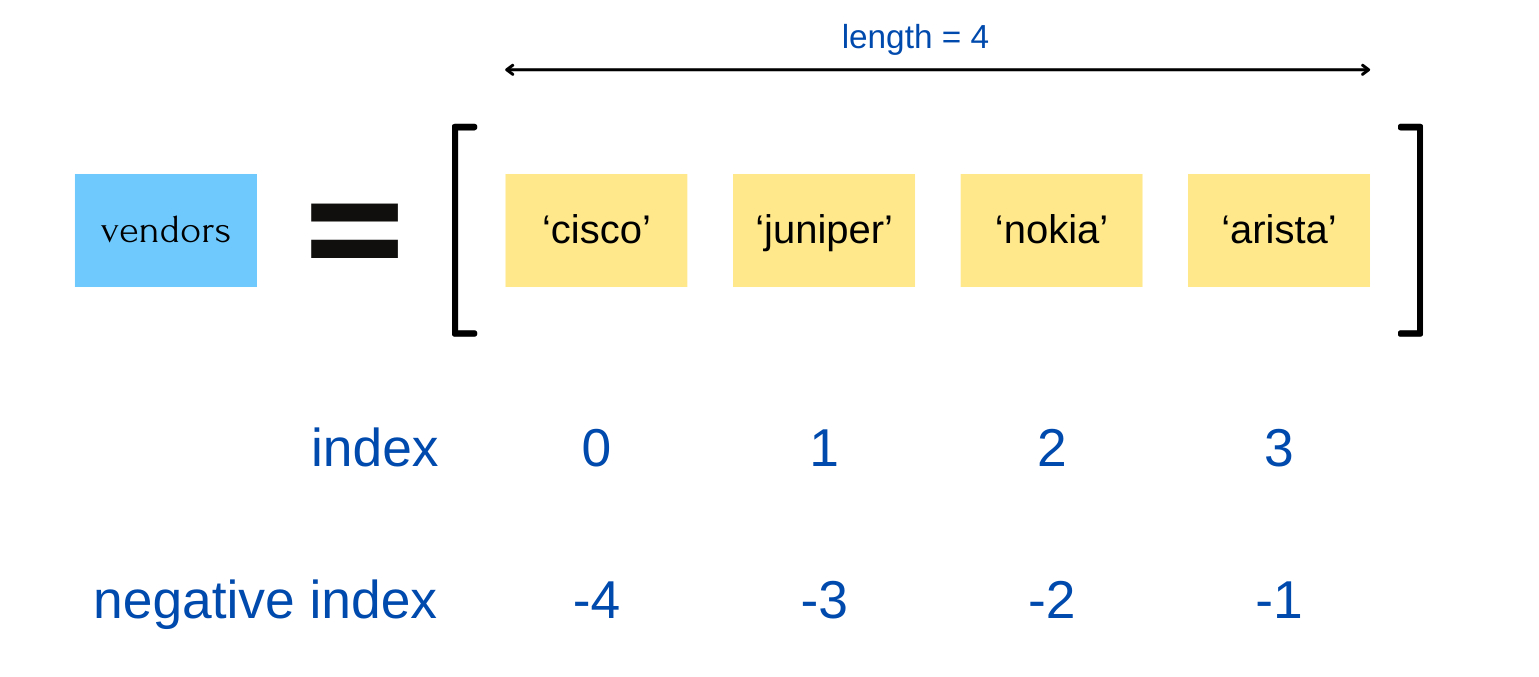
Accessing items in a Python list using their index is straightforward and efficient. Each item in a list is assigned a specific position or index, which starts from 0
for the first item, 1
for the second, and so on. You can use these indexes to extract specific items directly. To access an item, you simply specify the list name followed by the index of the item you want in square brackets.
ip_addresses = ['192.168.1.1', '10.0.0.1', '172.16.0.1']
device_names = ['Router1', 'Switch1', 'Firewall1']
- To access the first IP address in the
ip_addresses
list, you useip_addresses[0]
, which will give you'192.168.1.1'
. - To get the second device name in the
device_names
list, usedevice_names[1]
, resulting in'Switch1'
. - Python also allows you to use negative indexes to access items from the end of the list. For example, an index of
-1
refers to the last item,-2
to the second last, and so on. ip_addresses[-1]
would give you'172.16.0.1'
, the last IP address in the list.device_names[-2]
would return'Switch1'
, the second last device name.
Adding items to list
To add items to the device_names
list, you can use the append()
method to add a single item or the extend()
method to add multiple items from another list.
device_names.append('AccessPoint1')
#list will now include the AccessPoint1 we just added
device_names = ['Router1', 'Switch1', 'Firewall1', 'AccessPoint1']
You can also use the extend()
method to add multiple items from a different list.
new_devices = ['Router2', 'Switch2']
device_names.extend(new_devices)
#now list will include the new devices
device_names = ['Router1', 'Switch1', 'Firewall1', 'AccessPoint1', 'Router2', 'Switch2']
Tuples
Tuples are ordered collections like lists, but they are immutable, meaning once a tuple is created, its contents cannot be changed. Tuples are denoted by parentheses ()
.
device_properties = ('Cisco', 'Router', 'IOS-XE')
When we say that tuples are "immutable," it means that once a tuple is created, its contents cannot be changed, unlike lists. You can't add, remove, or alter items in a tuple after it's defined. This property makes tuples useful for storing data that shouldn't be modified through the course of a program.
Accessing items in a tuple works the same way as in a list, using indexes within square brackets.
- To access the first item (the manufacturer), you use
device_properties[0]
, which gives you'Cisco'
. - To get the device type, use
device_properties[1]
, resulting in'Router'
.
Just like lists, tuples support both positive indexes (starting from 0) and negative indexes (counting backward from the end, with -1 being the last item). So, device_properties[-1]
would access the last item, 'IOS-XE'
, in this tuple.
Sets
Sets are unordered collections of unique items, meaning no duplicates are allowed. They are great for operations involving membership testing, removing duplicates, and mathematical operations like unions and intersections. Sets are denoted by curly braces {}
. In networking, you might use a set to ensure a list of IP addresses is unique.
unique_ips = {'192.168.1.1', '10.0.0.1', '192.168.1.1'}
Sets being "unordered" means that the items in a set do not have a fixed position; there is no sequence or order in which they are stored or appear. When you add items to a set or iterate over a set, the elements may appear in any order, and this order can change with every execution of your program. This characteristic distinguishes sets from lists and tuples, which are ordered collections.
Accessing items directly in a set by index isn't possible because sets are unordered collections. However, you can still perform operations that don't rely on order. For example, you can check if a specific item is present in a set (membership testing), add new items, or remove items.
Dictionaries
Dictionaries are unordered collections of key-value pairs, allowing you to store and retrieve data with a key. They are denoted by curly braces {}
, with key-value pairs separated by colons :
. Dictionaries are perfect for storing device configurations or network state information where each piece of data has a specific label.
device_config = {
'hostname': 'Router1',
'ip_address': '192.168.1.1',
'os_version': 'IOS-XE 17.3.2',
'interfaces': ['GigabitEthernet0/0', 'GigabitEthernet0/1']
}
Working with dictionaries in Python is straightforward. Here's how to add a new key/value pair, change an existing value, and access a specific value using the device_config
dictionary as an example.
To add a new key/value pair to a dictionary, simply assign a value to a new key like so.
device_config['location'] = 'DataCenterA'
Here is how to change the value associated with an existing key, assign a new value to that key.
device_config['os_version'] = 'IOS-XE 17.4.1'
To access the value for a specific key, use the key inside square brackets as shown below.
print(device_config['hostname'])
This prints the value associated with 'hostname'
, which is 'Router1'
Why do I need all of these different data types?
You might be wondering why you need to learn about all these different data types like lists, dictionaries, and sets in Python. Let's consider a practical scenario where you're automating the creation of VLANs across multiple switches in a network.
You start by collecting the IP addresses of all the switches you need to configure and store them in a list. However, with hundreds of switches, there's a chance some IP addresses might be repeated. To ensure each IP is unique, you can convert your list into a set, which automatically removes any duplicates. After cleaning up the list, you then convert it back to a list format for further processing.
For the VLAN configurations, you'll need a way to associate each VLAN name with its corresponding VLAN ID. This is where a dictionary comes in handy, allowing you to pair each VLAN name (a string) with its VLAN ID (an integer) as key-value pairs. This makes your data organized and easily accessible for automation scripts.
# Initial list of switch IP addresses, potentially with duplicates
switch_ips = ['192.168.1.1', '192.168.1.2', '192.168.1.1', '192.168.1.3']
# Remove duplicates by converting the list to a set, then back to a list
unique_ips = list(set(switch_ips))
# Dictionary to map VLAN names to VLAN IDs
vlan_config = {'Sales': 10, 'Engineering': 20, 'Management': 30}
Summary
Let's bring together everything we've learned about basic and collection data types with a straightforward example. Imagine you're a network engineer who needs to keep track of various network devices, their types, IP addresses, and whether they're currently active (online or offline).
First, we'll use strings and booleans for device names and status. Then, we'll incorporate integers or floats for something like a device's uptime in hours. Finally, we'll utilize lists, tuples, sets, and dictionaries to organize this data.
# Basic Data Types
device_name = "Router1" # string
device_online = True # boolean
uptime_hours = 36 # integer
print(device_name, "online:", device_online, "for", uptime_hours, "hours")
# Collection Data Types
# List of IP addresses assigned to Router1
ip_addresses = ['192.168.1.1', '10.0.0.2'] # list
# Tuple for hardware specs (model, Ports, PSU)
hardware_specs = ('C9300', '48', 'Dual') # tuple
# Set of unique network protocols supported by Router1
network_protocols = {'HTTP', 'FTP', 'SSH'} # set
# Dictionary for Router1 configuration
router1_config = { # dictionary
'hostname': 'Router1',
'ip_addresses': ip_addresses,
'hardware_specs': hardware_specs,
'protocols': network_protocols,
'status': 'online'
}
print(router1_config)
- We start with basic data types to store single pieces of information about our network device.
- Then, we use a list to handle multiple IP addresses since a device can have more than one.
- A tuple is perfect for the hardware specs because these specs won't change once the device is deployed.
- A set ensures that the network protocols listed are unique, with no duplicates.
- Finally, a dictionary organizes all this data about Router1, making it easy to access any specific piece of information.
This example demonstrates how combining different data types allows us to model complex real-world data efficiently. As you continue to learn Python, think about how you can use these data types to represent and solve problems in your network engineering tasks.
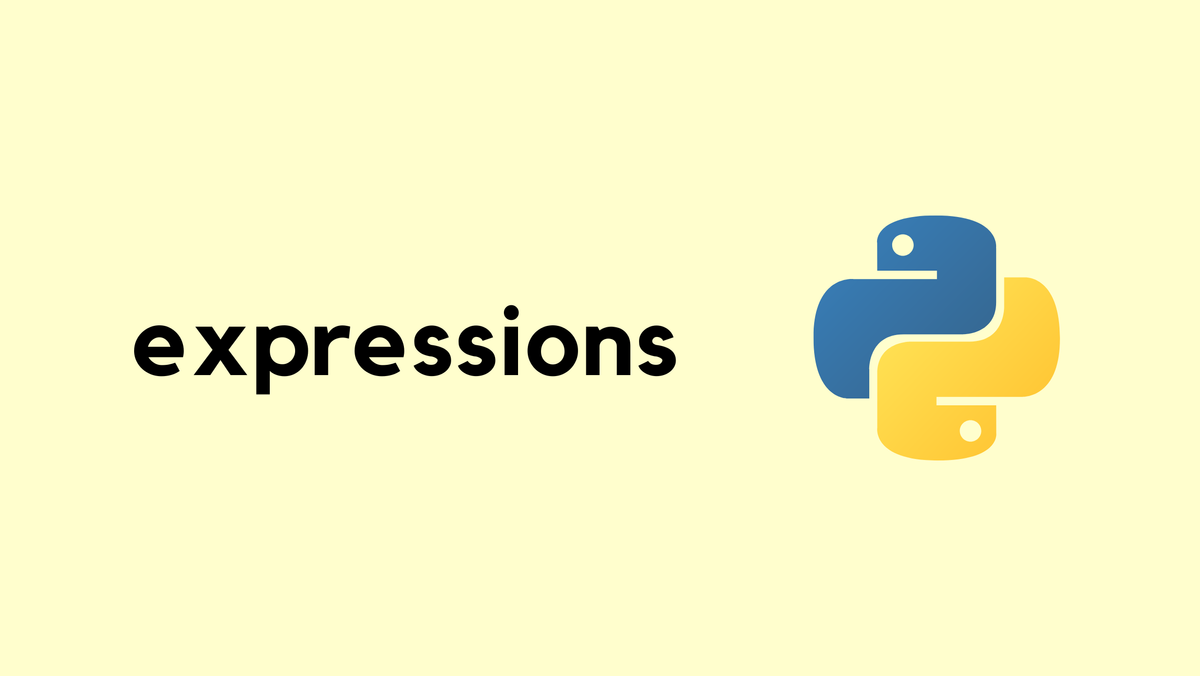